使用c++ 在Linux环境下用结构体写出CSize类的替代品 并写出所有的方法
时间: 2024-03-12 19:43:28 浏览: 12
好的,我理解您的问题了。为了解决问题,我们需要定义一个名为`CSize`的结构体,该结构体包含两个成员变量`width`和`height`,用来表示大小的宽度和高度。我们可以在结构体中定义一些方法来模拟类的行为。以下是一个示例:
```c++
#include <iostream>
struct CSize {
int width;
int height;
CSize(int w, int h) : width(w), height(h) {}
CSize() : CSize(0, 0) {}
void SetSize(int w, int h) {
width = w;
height = h;
}
bool operator==(const CSize& size) const {
return width == size.width && height == size.height;
}
bool operator!=(const CSize& size) const {
return !(*this == size);
}
CSize operator+(const CSize& size) const {
return CSize(width + size.width, height + size.height);
}
CSize operator-(const CSize& size) const {
return CSize(width - size.width, height - size.height);
}
CSize operator*(float scale) const {
return CSize(static_cast<int>(width * scale), static_cast<int>(height * scale));
}
CSize operator/(float scale) const {
return CSize(static_cast<int>(width / scale), static_cast<int>(height / scale));
}
CSize& operator+=(const CSize& size) {
width += size.width;
height += size.height;
return *this;
}
CSize& operator-=(const CSize& size) {
width -= size.width;
height -= size.height;
return *this;
}
CSize& operator*=(float scale) {
width = static_cast<int>(width * scale);
height = static_cast<int>(height * scale);
return *this;
}
CSize& operator/=(float scale) {
width = static_cast<int>(width / scale);
height = static_cast<int>(height / scale);
return *this;
}
friend std::ostream& operator<<(std::ostream& os, const CSize& size) {
os << "CSize(" << size.width << ", " << size.height << ")";
return os;
}
};
int main() {
CSize size1(100, 200);
CSize size2(50, 50);
std::cout << "Size1: " << size1 << std::endl;
std::cout << "Size2: " << size2 << std::endl;
size1 += size2;
std::cout << "Size1 after += Size2: " << size1 << std::endl;
CSize size3 = size1 * 0.5f;
std::cout << "Size3 = Size1 * 0.5f: " << size3 << std::endl;
return 0;
}
```
上面的代码定义了一个`CSize`结构体,它包含了一些常用方法,如`SetSize`、`operator==`、`operator+`等等。我们可以通过这些方法来修改和比较`CSize`对象。在`main`函数中,我们创建了两个`CSize`对象`size1`和`size2`,并执行了一些操作,如`size1 += size2`,`size3 = size1 * 0.5f`等等。
这只是一个简单的示例,您可以根据需要添加更多的方法和运算符重载来实现更复杂的功能。
相关推荐
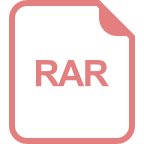
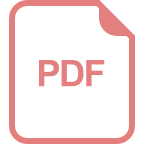
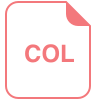
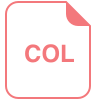
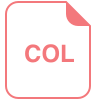
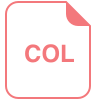
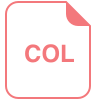









