sql with 案例
时间: 2023-09-22 10:06:15 浏览: 196
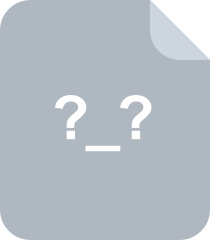
sql server 案例
### 回答1:
Sure, here is an SQL case study for a fictitious e-commerce website called "MyShop":
MyShop has the following tables:
- Customers: contains customer information such as name, email, phone number, etc.
- Orders: contains order information such as order date, customer ID, total price, etc.
- Order_Items: contains details of each item in an order such as product ID, quantity, price, etc.
- Products: contains product information such as name, description, price, etc.
Let's assume we want to answer the following business questions using SQL:
1. What is the total revenue generated by the website in the last month?
2. Who are the top 10 customers in terms of total spending on the website?
3. Which products are the most popular among customers?
Here are the SQL queries to answer these questions:
1. What is the total revenue generated by the website in the last month?
```sql
SELECT SUM(oi.quantity * p.price) as total_revenue
FROM Orders o
JOIN Order_Items oi ON o.order_id = oi.order_id
JOIN Products p ON oi.product_id = p.product_id
WHERE o.order_date >= DATEADD(month, -1, GETDATE())
```
This query joins the Orders, Order_Items, and Products tables to get the quantity and price of each item in an order and then sums up the revenue for all orders in the last month.
2. Who are the top 10 customers in terms of total spending on the website?
```sql
SELECT c.customer_id, c.name, SUM(oi.quantity * p.price) as total_spending
FROM Customers c
JOIN Orders o ON c.customer_id = o.customer_id
JOIN Order_Items oi ON o.order_id = oi.order_id
JOIN Products p ON oi.product_id = p.product_id
GROUP BY c.customer_id, c.name
ORDER BY total_spending DESC
LIMIT 10
```
This query joins the Customers, Orders, Order_Items, and Products tables to get the total spending for each customer and then sorts the result by total spending in descending order and limits the result to the top 10 customers.
3. Which products are the most popular among customers?
```sql
SELECT p.product_id, p.name, SUM(oi.quantity) as total_quantity_sold
FROM Products p
JOIN Order_Items oi ON p.product_id = oi.product_id
GROUP BY p.product_id, p.name
ORDER BY total_quantity_sold DESC
LIMIT 10
```
This query joins the Products and Order_Items tables to get the total quantity sold for each product and then sorts the result by total quantity sold in descending order and limits the result to the top 10 products.
### 回答2:
SQL with是一种使用SQL语句和子查询来创建临时视图的方法,以便更轻松地对数据进行操作和分析。下面我将用一个案例来阐述如何使用SQL with。
假设我们有一个电子商务网站的数据库,其中包含了用户表(User)、订单表(Order)和商品表(Product)。现在我们想要查询某个用户的订单信息,并显示每个订单的商品数量和总价。
首先,我们可以使用SQL with语句来创建一个临时视图,将用户表、订单表和商品表连接在一起。具体的SQL语句如下:
WITH Temp AS
(
SELECT
User.username,
Order.order_id,
Order.product_id,
Order.quantity,
Product.price
FROM
User
INNER JOIN Order ON User.user_id = Order.user_id
INNER JOIN Product ON Order.product_id = Product.product_id
)
在这个SQL with语句中,我们将用户表、订单表和商品表连接在一起,并将结果存储在名为Temp的临时视图中。在临时视图中,我们选择了用户表的用户名(User.username)、订单表的订单号(Order.order_id)、商品表的商品号(Order.product_id)、订单表的商品数量(Order.quantity)和商品表的商品价格(Product.price)。
接下来,我们可以使用创建的临时视图Temp来查询某个用户的订单信息,并计算每个订单的商品数量和总价。具体的SQL语句如下:
SELECT
Temp.username,
Temp.order_id,
SUM(Temp.quantity) AS total_quantity,
SUM(Temp.quantity * Temp.price) AS total_price
FROM
Temp
WHERE
Temp.username = '某个用户'
GROUP BY
Temp.order_id
在这个查询语句中,我们选择了临时视图Temp中的用户名(Temp.username)、订单号(Temp.order_id),并使用SUM函数来计算每个订单的商品数量(SUM(Temp.quantity))和总价(SUM(Temp.quantity * Temp.price))。最后,我们使用WHERE子句来限制查询结果只包含某个特定用户的订单,并使用GROUP BY子句按订单号进行分组。
通过使用SQL with语句和临时视图,我们可以更方便地对数据进行操作和分析,减少了编写复杂查询语句的复杂度。
### 回答3:
SQL with是一种在SQL语句中定义临时表格的功能。它能够方便地在执行复杂查询时创建临时表格,提高查询的效率和易读性。
假设有一个学生表格(Student)和一个成绩表格(Score),我们需要查询每个学生的平均成绩以及平均分大于80分的学生姓名和平均分数。
使用SQL with可以很方便地实现这个查询:
```
WITH avg_score AS (
SELECT student_id, AVG(score) AS average_score
FROM score
GROUP BY student_id
)
SELECT s.name, a.average_score
FROM student s
JOIN avg_score a ON s.id = a.student_id
WHERE a.average_score > 80;
```
在这个查询中,我们首先定义了一个名为avg_score的临时表格,它包含了每个学生的平均成绩。然后我们再将该临时表格与学生表格进行关联,筛选出平均分大于80分的学生姓名和平均分数。
通过使用SQL with,可以将查询过程分解为多个步骤,使得查询更易于理解和维护。此外,临时表格的使用还能够提高查询的执行效率,特别是当需要多次使用同一临时表格时。
总之,SQL with是一个非常有用的功能,它可以提高SQL查询的可读性和执行效率,使得复杂查询更容易理解和维护。
阅读全文
相关推荐
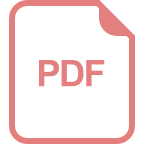
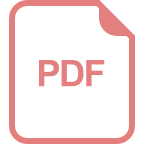
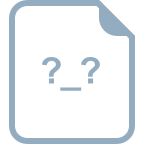
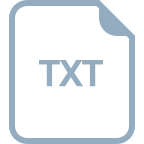
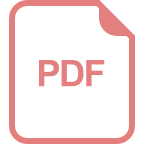
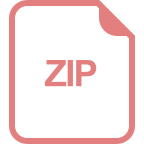
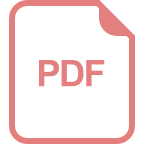
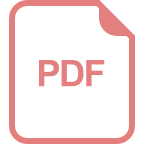
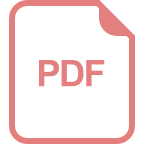
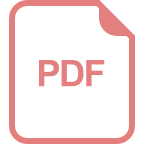
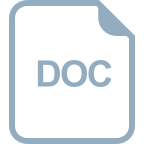
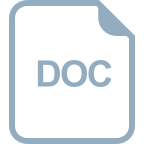
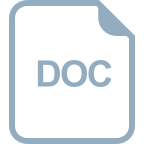
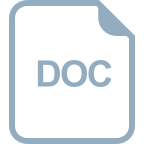
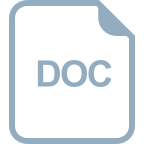