使用mybatis-plus怎么写管理功能
时间: 2023-06-19 15:05:02 浏览: 86
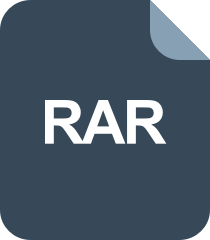
mybatis-plus基础功能实现
使用Mybatis-Plus编写管理功能可以分为以下几个步骤:
1. 创建实体类
首先需要创建对应的实体类,可以使用Lombok注解来简化代码。例如:
```
@Data
@EqualsAndHashCode(callSuper = false)
@Accessors(chain = true)
public class User implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 主键ID
*/
@TableId(value = "id", type = IdType.AUTO)
private Long id;
/**
* 用户名
*/
private String username;
/**
* 密码
*/
private String password;
/**
* 创建时间
*/
@TableField(fill = FieldFill.INSERT)
private Date createTime;
/**
* 修改时间
*/
@TableField(fill = FieldFill.INSERT_UPDATE)
private Date updateTime;
}
```
2. 创建Mapper接口
使用Mybatis-Plus可以省略编写Mapper接口的过程,直接使用BaseMapper即可。例如:
```
public interface UserMapper extends BaseMapper<User> {
}
```
3. 创建Service
创建Service层,用于实现具体的业务逻辑。例如:
```
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
}
```
4. 创建Controller
创建Controller层,用于接收请求并处理业务逻辑。例如:
```
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping
public List<User> list() {
return userService.list();
}
@PostMapping
public boolean save(@RequestBody User user) {
return userService.save(user);
}
@PutMapping
public boolean update(@RequestBody User user) {
return userService.updateById(user);
}
@DeleteMapping("/{id}")
public boolean remove(@PathVariable Long id) {
return userService.removeById(id);
}
}
```
以上就是使用Mybatis-Plus编写管理功能的基本步骤。需要注意的是,在实体类中使用注解@TableId、@TableField等来指定数据库表的主键和字段映射关系;在Controller层中使用注解@GetMapping、@PostMapping、@PutMapping、@DeleteMapping等来指定请求的方法和路径。
阅读全文
相关推荐
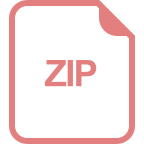
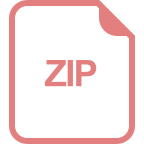
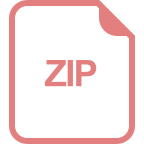
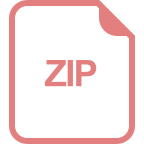
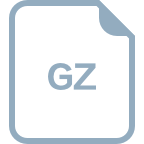
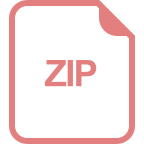
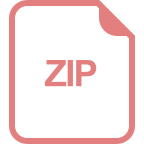
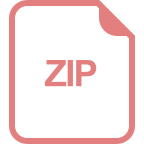
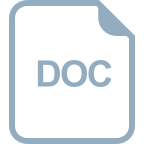
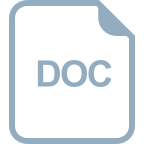
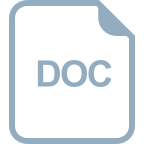
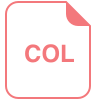
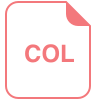
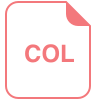



