c语言怎么进行日期比较
时间: 2024-10-07 14:04:25 浏览: 57
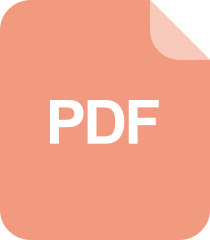
C语言计算日期差的方法示例
在C语言中,比较日期通常涉及到时间戳或者是结构体表示日期,因为C标准本身并不提供直接操作日期的内置函数。这里我们可以使用`time_t`类型的时间戳来进行比较,或者自定义日期结构体并实现相应的比较方法。以下是两种常见的做法:
1. **使用时间戳**:
- `time()`函数获取当前时间的Unix时间戳(秒数自从1970年1月1日00:00:00 GMT以来的秒数)。如果需要比较两个日期,可以直接比较这两个时间戳的值。
- `difftime(time1, time2)` 函数计算两个时间戳之间的差值(以秒为单位),用于判断两个日期先后顺序。
```c
#include <stdio.h>
#include <time.h>
int main() {
time_t t1 = time(NULL); // 获取当前时间
time_t t2 = /* 你需要比较的具体时间 */;
if (t1 > t2) {
printf("t1 is later than t2.\n");
} else if (t1 < t2) {
printf("t1 is earlier than t2.\n");
} else {
printf("t1 and t2 are the same time.\n");
}
}
```
2. **自定义日期结构**:
- 定义一个包含年、月、日三个字段的结构体,如`struct date`,然后为其提供比较运算符(如`<`, `>`, `==`)。
```c
struct date {
int year;
int month;
int day;
};
// 比较两个日期结构
int compare_dates(const struct date* d1, const struct date* d2) {
return (d1->year * 10000 + d1->month * 100 + d1->day) -
(d2->year * 10000 + d2->month * 100 + d2->day);
}
// 然后在主程序里这样比较
struct date date1 = {2023, 1, 1};
struct date date2 = {2022, 12, 31};
if (compare_dates(&date1, &date2) > 0) {
printf("date1 is later than date2.\n");
}
```
阅读全文
相关推荐
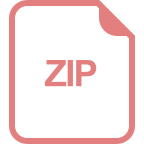
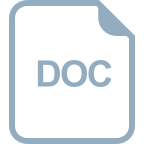
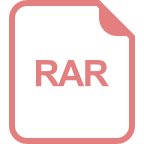
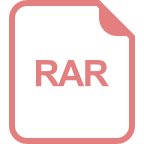
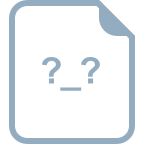
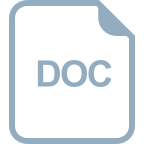
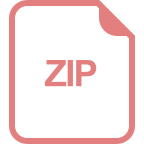
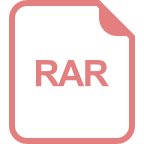
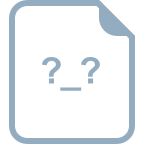
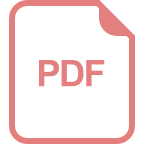
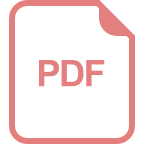
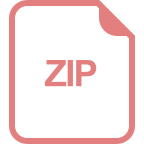
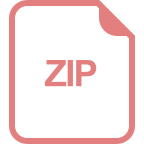
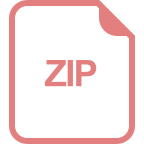