Linux删除链表中的元素
时间: 2024-05-02 11:21:51 浏览: 7
要在Linux中删除链表中的元素,您需要使用C或C ++编程语言来实现。以下是一些步骤:
1. 首先,您需要定义一个链表结构体,该结构体包含一个指向下一个节点的指针和一个值。
2. 然后,创建一个指向链表头的指针。
3. 使用循环遍历链表,直到找到需要删除的节点。
4. 在找到节点后,将其前一个节点的指针指向其后一个节点,然后释放该节点的内存。
5. 如果需要删除链表的第一个节点,则将链表头指针更新为链表的下一个节点。
6. 最后,释放链表头指针的内存。
以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int value;
struct node* next;
};
void deleteNode(struct node** head, int key) {
struct node* currentNode = *head;
struct node* previousNode = NULL;
if (currentNode != NULL && currentNode->value == key) {
*head = currentNode->next;
free(currentNode);
return;
}
while (currentNode != NULL && currentNode->value != key) {
previousNode = currentNode;
currentNode = currentNode->next;
}
if (currentNode == NULL) {
return;
}
previousNode->next = currentNode->next;
free(currentNode);
}
int main() {
struct node* head = NULL;
struct node* second = NULL;
struct node* third = NULL;
head = (struct node*)malloc(sizeof(struct node));
second = (struct node*)malloc(sizeof(struct node));
third = (struct node*)malloc(sizeof(struct node));
head->value = 1;
head->next = second;
second->value = 2;
second->next = third;
third->value = 3;
third->next = NULL;
deleteNode(&head, 2);
struct node* currentNode = head;
while (currentNode != NULL) {
printf("%d ", currentNode->value);
currentNode = currentNode->next;
}
return 0;
}
```
在这个示例中,我们定义了一个`node`结构体,其中包含一个`value`字段和一个`next`指针,该指针指向下一个节点。我们还定义了一个`deleteNode`函数,该函数使用循环遍历链表并删除特定的节点。在`main`函数中,我们创建一个包含三个节点的链表,并调用`deleteNode`函数来删除第二个节点。最后,我们打印出更新后的链表。
相关推荐
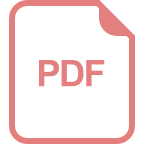
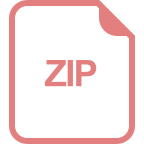














