将若干城市的信息存人一个带头结点的单链表,结点中的城市信息包括城市名、 城市的位置坐标。要求:C语言 (1)给定一个城市名,返回其位置坐标; (2)给定一个位置坐标P和一个距离D,返回所有与P的距离小于等于D的城市。
时间: 2024-09-30 16:03:23 浏览: 49
在C语言中,你可以设计一个结构体表示城市信息,并创建一个链表来存储这些城市。首先,定义一个`City`结构体,包含城市名`name`和位置坐标`(x, y)`:
```c
typedef struct {
char name[50]; // 假设最大城市名长度为49字符,留一位空间给'\0'
float x, y; // 位置坐标
} City;
```
接着,创建一个单链表节点`CityNode`,包含城市信息和指向下一个城市的指针:
```c
typedef struct {
City city;
CityNode* next;
} CityNode;
```
为了实现功能:
1. 给定城市名查找坐标:
- 定义一个辅助函数`find_city_by_name(CityList* list, const char* name)`,其中`CityList`是指向链表头节点的指针。遍历链表,如果找到匹配的城市名,返回它的坐标;如果没有找到,可以返回NULL。
```c
CityNode* find_city_by_name(CityList* list, const char* name) {
CityNode* current = list->head;
while (current != NULL) {
if (strcmp(current->city.name, name) == 0) {
return ¤t->city;
}
current = current->next;
}
return NULL;
}
```
2. 给定坐标和距离查询城市:
- 定义`get_cities_within_distance(CityList* list, float x, float y, float D)`。同样遍历链表,计算每个城市的距离并判断是否小于等于D,将满足条件的城市添加到结果列表或其他数据结构中。
```c
void get_cities_within_distance(CityList* list, float x, float y, float D, CityNode** result) {
CityNode* current = list->head;
while (current != NULL) {
float distance = sqrt(pow(current->city.x - x, 2) + pow(current->city.y - y, 2));
if (distance <= D) {
*result = add_city_to_result(*result, current);
}
current = current->next;
}
}
CityNode* add_city_to_result(CityNode* result, CityNode* new_city) {
// 如果result为空,直接新建一个链表
if (result == NULL) {
result = malloc(sizeof(CityNode));
result->city = new_city->city;
result->next = NULL;
} else {
// 否则追加到现有链表
CityNode* temp = result;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = malloc(sizeof(CityNode));
temp->next->city = new_city->city;
temp->next->next = NULL;
}
return result;
}
```
阅读全文
相关推荐












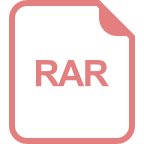




