用python写一个将当前系统时间写入指定路径的文件中的例子
时间: 2024-03-02 20:50:35 浏览: 59
好的,以下是一个用 Python 实现的将当前系统时间写入指定路径文件的例子:
```python
import os
import datetime
# 定义要写入的文件路径
file_path = '/path/to/file.txt'
# 获取当前系统时间
now = datetime.datetime.now()
# 将时间格式化为字符串
time_str = now.strftime('%Y-%m-%d %H:%M:%S')
# 将时间写入指定文件中
with open(file_path, 'w') as f:
f.write(time_str)
# 打印写入成功的提示信息
print('当前系统时间已写入文件:', os.path.abspath(file_path))
```
在运行这段代码之前,需要将 `file_path` 变量替换为你要写入的文件路径。该代码会获取当前系统时间,将其格式化为字符串并写入指定的文件中。最后,会打印写入成功的提示信息。
相关问题
python文件操作,将单词写入当前路径下的一个新文件中
假设你已经有一个字符串变量 `content`,其中包含了需要写入文件的单词。你可以使用 Python 的内置函数 `open()` 和 `write()` 来将这些单词写入一个新文件。
```python
content = "apple banana orange"
with open("words.txt", "w") as file:
file.write(content)
```
在这个例子中,我们打开一个名为 `words.txt` 的文件,并将 `content` 字符串写入到文件中。`'w'` 表示以写入模式打开文件,如果文件不存在则会创建它。使用 `with` 语句可以自动关闭文件,避免了手动调用 `close()` 函数。
如果你想将单词写入文件的同时,每个单词占一行,可以将 `content` 字符串中的单词用换行符 `\n` 分隔开来:
```python
content = "apple\nbanana\norange"
with open("words.txt", "w") as file:
file.write(content)
```
这样,每个单词就会被写入文件的一行中。
python 如何将文件写入到指定文件夹中
要文件写入到指定文件夹中,您可以使用Python的`os`和`shutil`模块。
以下是一个示例代码:
```python
import os
import shutil
source_file = '/path/to/source/file.txt'
destination_folder = '/path/to/destination/folder'
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
shutil.copy(source_file, destination_folder)
```
在这个例子中,我们首先指定了源文件的路径`source_file`和目标文件夹的路径`destination_folder`。然后我们使用`os.makedirs()`函数创建目标文件夹(如果它不存在)。最后,我们使用`shutil.copy()`函数将源文件复制到目标文件夹中。
请注意,`shutil.copy()`函数将复制源文件并将其放置在目标文件夹中。如果您希望移动源文件而不是复制它,请使用`shutil.move()`函数。
阅读全文
相关推荐
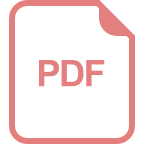












