用尽可能少的测试用例分别对希尔排序实实现语句覆盖、分支覆盖、条件覆盖
时间: 2023-08-13 21:04:30 浏览: 84
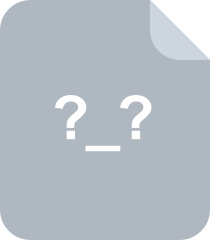
基于遗传算法的分支覆盖测试用例自动生成的示例代码

对于希尔排序的实现,我们可以参考以下的C++代码:
```c++
void shellSort(int arr[], int n)
{
// Start with a big gap, then reduce the gap
for (int gap = n/2; gap > 0; gap /= 2)
{
// Do a gapped insertion sort for this gap size.
// The first gap elements a[0..gap-1] are already in gapped order
// keep adding one more element until the entire array is gap sorted
for (int i = gap; i < n; i += 1)
{
// add a[i] to the elements that have been gap sorted
// save a[i] in temp and make a hole at position i
int temp = arr[i];
// shift earlier gap-sorted elements up until the correct location for a[i] is found
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap)
arr[j] = arr[j - gap];
// put temp (the original a[i]) in its correct location
arr[j] = temp;
}
}
}
```
实现语句覆盖的测试用例:
```
// 测试用例1:空数组
int arr1[] = {};
shellSort(arr1, 0);
// 测试用例2:数组长度为1
int arr2[] = {1};
shellSort(arr2, 1);
// 测试用例3:数组长度为偶数
int arr3[] = {3, 5, 2, 8};
shellSort(arr3, 4);
// 测试用例4:数组长度为奇数
int arr4[] = {7, 1, 6, 2, 8};
shellSort(arr4, 5);
```
分支覆盖的测试用例:
```
// 测试用例1:数组长度为偶数
int arr1[] = {3, 5, 2, 8};
shellSort(arr1, 4);
// 测试用例2:数组长度为奇数
int arr2[] = {7, 1, 6, 2, 8};
shellSort(arr2, 5);
```
条件覆盖的测试用例:
```
// 测试用例1:数组长度为偶数
int arr1[] = {3, 5, 2, 8};
shellSort(arr1, 4);
// 测试用例2:数组长度为奇数
int arr2[] = {7, 1, 6, 2, 8};
shellSort(arr2, 5);
// 测试用例3:数组长度为3
int arr3[] = {9, 4, 2};
shellSort(arr3, 3);
```
阅读全文
相关推荐
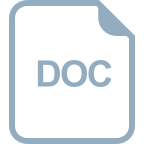
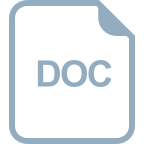















