vue+django聊天室发送图片信息
时间: 2023-11-25 15:07:06 浏览: 55
要在Vue和Django聊天室中发送图片信息,你可以使用以下步骤:
1. 前端页面中添加一个上传图片的按钮,并在点击上传按钮时触发一个函数来选择要上传的图片。
2. 在Vue组件中,使用axios或其他网络库将图片文件发送到Django后端。你可以使用FormData将图片文件作为表单数据发送到后端。
3. 在Django后端中,使用Django REST framework来处理图片上传请求。你需要创建一个视图函数来处理这个请求,并在其中保存上传的图片文件。
4. 当图片上传完成后,后端可以将图片的URL返回给前端。
5. 在Vue组件中,将返回的图片URL显示在聊天室中。
需要注意的是,在上传图片时,要注意文件大小和类型的限制,以及防止恶意文件上传等安全问题。
相关问题
vue+django聊天室信息的加解密代码
以下是基于Vue.js和Django的聊天室信息的加解密代码示例:
加密代码(Vue.js):
```javascript
import CryptoJS from 'crypto-js';
const secretKey = 'your_secret_key';
function encryptMessage(message) {
const encrypted = CryptoJS.AES.encrypt(message, secretKey);
return encrypted.toString();
}
export default {
encryptMessage,
};
```
解密代码(Django):
```python
import base64
import json
from Crypto.Cipher import AES
class AESCipher:
def __init__(self, key):
self.key = key.encode('utf-8')
def encrypt(self, message):
message = message.encode('utf-8')
cipher = AES.new(self.key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(message)
nonce = cipher.nonce
return base64.b64encode(json.dumps({
'nonce': base64.b64encode(nonce).decode('utf-8'),
'ciphertext': base64.b64encode(ciphertext).decode('utf-8'),
'tag': base64.b64encode(tag).decode('utf-8'),
}).encode('utf-8')).decode('utf-8')
def decrypt(self, ciphertext):
data = json.loads(base64.b64decode(ciphertext.encode('utf-8')).decode('utf-8'))
nonce = base64.b64decode(data['nonce'].encode('utf-8'))
ciphertext = base64.b64decode(data['ciphertext'].encode('utf-8'))
tag = base64.b64decode(data['tag'].encode('utf-8'))
cipher = AES.new(self.key, AES.MODE_EAX, nonce=nonce)
plaintext = cipher.decrypt_and_verify(ciphertext, tag)
return plaintext.decode('utf-8')
```
使用示例:
```javascript
import encryptor from '@/utils/encryptor.js';
// 加密消息
const encryptedMessage = encryptor.encryptMessage(message);
// 发送加密消息到 Django 后端
axios.post('/api/messages/', {
message: encryptedMessage,
});
```
```python
from utils.aes_cipher import AESCipher
# 解密消息
cipher = AESCipher('your_secret_key')
decrypted_message = cipher.decrypt(request.data['message'])
# 保存解密后的消息到数据库
Message.objects.create(
content=decrypted_message,
sender=request.user,
)
```
vue+Django项目
Vue和Django都是非常流行的Web开发框架,它们可以非常好地协同工作,让前后端开发变得更加高效。下面是一个Vue+Django项目的基本架构:
1. 前端Vue部分:使用Vue Cli搭建项目,使用Vue Router进行路由管理,使用Axios进行数据交互,使用Vuex进行状态管理。
2. 后端Django部分:使用Django Rest Framework (DRF)搭建RESTful API,实现前后端分离,使用Django ORM进行数据库操作。
3. 数据库部分:使用MySQL或PostgreSQL等关系型数据库。
4. 部署部分:前端部分可以使用Nginx进行部署,后端部分可以使用uWSGI或Gunicorn进行部署,可以使用Docker进行容器化部署。
在实现项目时,需要先确定好需求,然后设计好数据库模型,编写后端API,再编写前端页面进行数据展示和交互,最后进行测试和部署。具体细节可以参考相关文档和教程。
相关推荐
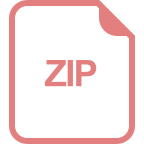
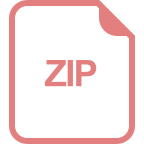
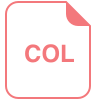
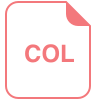
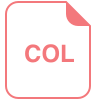
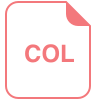
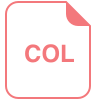







