能把上述程序转化为基于Matlab实现吗
时间: 2024-02-13 15:00:45 浏览: 111
当然可以,以下是一个基于Matlab实现的使用遗传算法解决多配送中心选址问题的示例代码。
```matlab
% 定义遗传算法的参数
POP_SIZE = 50; % 种群数量
DNA_SIZE = 20; % 基因长度
CROSS_RATE = 0.8; % 交叉概率
MUTATION_RATE = 0.003; % 突变概率
N_GENERATIONS = 200; % 迭代次数
% 定义问题的参数
N_CENTERS = 5; % 配送中心数量
N_CUSTOMERS = 100; % 客户数量
MAX_DISTANCE = 100; % 最大距离
CUSTOMER_POSITIONS = randi(MAX_DISTANCE, [N_CUSTOMERS, 2]); % 生成客户的位置
% 定义适应度函数,用于评估每个个体的适应度
function f = fitness(population)
global N_CENTERS N_CUSTOMERS CUSTOMER_POSITIONS
distances = zeros(size(population, 1), N_CENTERS, N_CUSTOMERS);
for i = 1:size(population, 1)
for j = 1:N_CENTERS
center_pos = population(i, (j-1)*2+1:j*2);
distances(i, j, :) = sqrt(sum((CUSTOMER_POSITIONS - center_pos).^2, 2));
end
end
min_distances = min(distances, [], 2);
f = sum(sum(min_distances, 3), 2);
end
% 定义选择函数,用于选择下一代的个体
function p = select(population, fitness)
[~, sorted_idx] = sort(-fitness);
sorted_population = population(sorted_idx, :);
fitness = fitness(sorted_idx);
cumsum_fitness = cumsum(fitness);
cumsum_fitness = cumsum_fitness / cumsum_fitness(end);
selected_idx = randsample(size(population, 1), POP_SIZE, true, cumsum_fitness);
p = sorted_population(selected_idx, :);
end
% 定义交叉函数,用于交叉两个个体的基因
function [c1, c2] = crossover(parent1, parent2)
if rand() < CROSS_RATE
cross_pos = randi(DNA_SIZE);
c1 = [parent1(1:cross_pos), parent2(cross_pos+1:end)];
c2 = [parent2(1:cross_pos), parent1(cross_pos+1:end)];
else
c1 = parent1;
c2 = parent2;
end
end
% 定义突变函数,用于突变个体的基因
function c = mutate(child)
for i = 1:length(child)
if rand() < MUTATION_RATE
child(i) = randi(MAX_DISTANCE);
end
end
c = child;
end
% 初始化种群
population = randi(MAX_DISTANCE, [POP_SIZE, N_CENTERS*2]);
% 迭代遗传算法
for generation = 1:N_GENERATIONS
% 计算每个个体的适应度
fitness_vals = fitness(population);
% 输出当前迭代的结果
fprintf("Generation: %d | Best Fitness: %f\n", generation, max(fitness_vals));
% 选择下一代的个体
population = select(population, fitness_vals);
% 交叉和突变,生成新的个体
for i = 1:(POP_SIZE/2)
parent1 = population(i*2-1, :);
parent2 = population(i*2, :);
[child1, child2] = crossover(parent1, parent2);
child1 = mutate(child1);
child2 = mutate(child2);
population(i*2-1, :) = child1;
population(i*2, :) = child2;
end
end
% 输出最终结果
[~, best_idx] = max(fitness_vals);
best_center_positions = reshape(population(best_idx, :), [N_CENTERS, 2]);
fprintf("Best Center Positions:\n");
disp(best_center_positions);
```
这个代码与之前的Python代码类似,使用遗传算法选择5个最佳的配送中心位置。在每次迭代中,使用适应度函数评估每个个体的适应度,然后使用选择、交叉和突变操作生成新的个体。最终输出最佳的配送中心位置。需要注意的是,Matlab中没有全局变量,需要在函数内部使用`global`关键字声明全局变量。
阅读全文
相关推荐

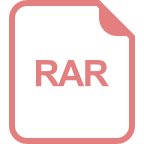





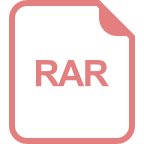


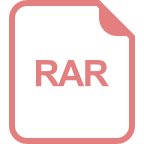




