vt.fit _transform
时间: 2023-07-21 09:38:57 浏览: 94
抱歉,我之前的回答有误。`vt.fit_transform`是sklearn中的`CountVectorizer`类的一个方法,用于将文本转换成特征向量,以便于机器学习算法的处理。CountVectorizer类可以对文本进行向量化处理,将文本中的单词转换为数字特征向量,以便于算法进行处理。`fit_transform`方法是CountVectorizer类中的一个函数,它将文本列表转换为特征向量,并返回转换后的结果。在转换时,该函数会先使用`fit`方法对数据进行拟合,然后再使用`transform`方法将数据进行转换。`fit_transform`方法实际上是将`fit`和`transform`两个方法进行了合并,可以直接进行拟合和转换操作。例如,对于一个文本列表text_list,可以使用以下代码进行向量化处理:
```
from sklearn.feature_extraction.text import CountVectorizer
vt = CountVectorizer()
text_vectorized = vt.fit_transform(text_list)
```
其中,CountVectorizer类首先会使用`fit`方法拟合text_list,得到单词的词典和编码方式,然后使用`transform`方法将text_list转换为特征向量的文本矩阵text_vectorized。上述代码中,`fit_transform`方法可以代替`fit`和`transform`方法,实现同样的功能。
相关问题
import numpy as np from tensorflow import keras from sklearn.decomposition import PCA import matplotlib.pyplot as plt (train_data, train_labels), (test_data, test_labels) = keras.datasets.mnist.load_data() Data_Vectorize = train_data.reshape(60000,784) N = 1000; index = np.where(train_labels==0)[0] index_0 = index[0:N] Data_0_Vectorize = Data_Vectorize[index_0] index = np.where(train_labels==1)[0] index_1 = index[0:N] Data_1_Vectorize = Data_Vectorize[index_1] Data_01_Vectorize = np.zeros([2*N,784]) Data_01_Vectorize[:N,:] = Data_0_Vectorize Data_01_Vectorize[N:,:] = Data_1_Vectorize My_pca = PCA(n_components=3) Data_01_Vectorize_DR = My_pca.fit_transform(Data_01_Vectorize) plt.scatter(Data_01_Vectorize_DR[:,0],Data_01_Vectorize_DR[:,1]) plt.xlabel('PC1') plt.ylabel('PC2') plt.show()在该代码基础上,针对“0”“1”混合样本,在PC1-PC2构成的低维空间中进行高斯混合聚类。聚类总数设置为2。在PC1-PC2散点图基础上画出高斯混合聚类的中心和3倍方差组成的椭圆形边界。
好的,我来回答你的问题。
针对“0”“1”混合样本,在PC1-PC2构成的低维空间中进行高斯混合聚类,可以使用sklearn中的GaussianMixture模型。具体实现步骤如下:
首先,需要导入GaussianMixture模型:
```
from sklearn.mixture import GaussianMixture
```
接着,对数据进行高斯混合聚类:
```
gmm = GaussianMixture(n_components=2).fit(Data_01_Vectorize_DR[:, :2])
labels = gmm.predict(Data_01_Vectorize_DR[:, :2])
```
这里将聚类总数设置为2,使用fit方法对数据进行拟合,再使用predict方法对数据进行聚类。
接下来,画出高斯混合聚类的中心和3倍方差组成的椭圆形边界。可以使用以下代码实现:
```
from matplotlib.patches import Ellipse
fig = plt.figure()
ax = fig.add_subplot(111)
colors = ['red', 'blue']
for i in range(2):
ax.scatter(Data_01_Vectorize_DR[labels == i, 0], Data_01_Vectorize_DR[labels == i, 1], c=colors[i])
for pos, covar, w in zip(gmm.means_, gmm.covariances_, gmm.weights_):
U, s, Vt = np.linalg.svd(covar)
angle = np.degrees(np.arctan2(U[1, 0], U[0, 0]))
width, height = 2 * np.sqrt(3 * s)
ellip = Ellipse(xy=pos, width=width, height=height, angle=angle, alpha=w)
ax.add_artist(ellip)
ellip.set_facecolor('none')
ellip.set_edgecolor('black')
plt.xlabel('PC1')
plt.ylabel('PC2')
plt.show()
```
这里使用matplotlib库的Ellipse类,根据高斯混合模型的均值和协方差矩阵画出椭圆形边界。其中,U, s, Vt = np.linalg.svd(covar)是对协方差矩阵进行奇异值分解,np.degrees(np.arctan2(U[1, 0], U[0, 0]))是计算旋转角度,width, height = 2 * np.sqrt(3 * s)是计算椭圆形边界的宽度和高度。
最终,可以得到画出高斯混合聚类的中心和3倍方差组成的椭圆形边界的PC1-PC2散点图。
基于NDT算法写出python代码实现:1.将点云数据划分为若干个体素;2.对每个体素内的点云数据进行高斯分布拟合;3.计算每个体素内的点云数据与目标点云数据的匹配度;4.根据匹配度进行点云配准。
感谢您的提问。对于您的问题,我可以回答。基于NDT算法的python代码实现如下:
1.将点云数据划分为若干个体素:
```
import numpy as np
from sklearn.neighbors import KDTree
def voxel_downsample(points, voxel_size):
"""
将点云数据划分为若干个体素
:param points: 点云数据
:param voxel_size: 体素大小
:return: 体素化后的点云数据
"""
min_point = np.min(points, axis=0)
max_point = np.max(points, axis=0)
voxel_grid = np.ceil((max_point - min_point) / voxel_size)
voxel_grid = voxel_grid.astype(np.int32)
voxel_points, voxel_point_idx = [], []
for i in range(voxel_grid[0]):
for j in range(voxel_grid[1]):
for k in range(voxel_grid[2]):
voxel_min = min_point + np.array([i, j, k]) * voxel_size
voxel_max = voxel_min + voxel_size
idx = np.where((points[:, 0] >= voxel_min[0]) & (points[:, 0] < voxel_max[0]) &
(points[:, 1] >= voxel_min[1]) & (points[:, 1] < voxel_max[1]) &
(points[:, 2] >= voxel_min[2]) & (points[:, 2] < voxel_max[2]))[0]
if len(idx) > 0:
voxel_points.append(np.mean(points[idx], axis=0))
voxel_point_idx.append(idx)
return np.array(voxel_points), voxel_point_idx
```
2.对每个体素内的点云数据进行高斯分布拟合:
```
from scipy.optimize import minimize
def gauss_newton(x0, y, sigma, f, jacobian, max_iter=20):
"""
高斯牛顿法拟合高斯分布
:param x0: 初始参数
:param y: 数据
:param sigma: 数据权重
:param f: 模型函数
:param jacobian: 模型函数的雅可比矩阵
:param max_iter: 最大迭代次数
:return: 拟合后的参数
"""
x = x0
for i in range(max_iter):
J = jacobian(x, y)
r = f(x, y) - sigma
H = J.T.dot(np.diag(sigma ** 2)).dot(J)
g = J.T.dot(sigma ** 2).dot(r)
dx = np.linalg.solve(H, -g)
x += dx
if np.linalg.norm(dx) < 1e-6:
break
return x
def gaussian_model(x, y):
"""
高斯分布模型函数
:param x: 参数
:param y: 数据
:return: 模型值
"""
mu = x[:3]
sigma = np.diag(x[3:6])
det = np.linalg.det(sigma)
if det == 0:
return np.zeros(len(y))
else:
inv_sigma = np.linalg.inv(sigma)
diff = y - mu
exponent = np.exp(-0.5 * np.sum(diff.dot(inv_sigma) * diff, axis=1))
return exponent / np.sqrt((2 * np.pi) ** 3 * det)
def gaussian_jacobian(x, y):
"""
高斯分布模型函数的雅可比矩阵
:param x: 参数
:param y: 数据
:return: 雅可比矩阵
"""
mu = x[:3]
sigma = np.diag(x[3:6])
inv_sigma = np.linalg.inv(sigma)
diff = y - mu
exponent = np.exp(-0.5 * np.sum(diff.dot(inv_sigma) * diff, axis=1))
dmu = np.sum(diff.dot(inv_sigma), axis=0)
dsigma = -0.5 * inv_sigma.dot(diff.T).dot(diff).dot(inv_sigma)
return np.hstack((np.tile(exponent, (3, 1)).T * dmu, np.tile(exponent, (6, 1)).T * dsigma))
def fit_gaussian(points):
"""
对每个体素内的点云数据进行高斯分布拟合
:param points: 体素内的点云数据
:return: 高斯分布的均值和协方差矩阵
"""
x0 = np.hstack((np.mean(points, axis=0), np.std(points, axis=0)))
res = minimize(lambda x: np.sum((gaussian_model(x, points) - 1) ** 2), x0, method='trust-krylov',
jac=lambda x: 2 * (gaussian_model(x, points) - 1)[:, np.newaxis] * gaussian_jacobian(x, points),
options={'maxiter': 100})
mu = res.x[:3]
sigma = np.diag(res.x[3:6])
return mu, sigma
```
3.计算每个体素内的点云数据与目标点云数据的匹配度:
```
def compute_correspondences(source_points, target_points, source_normals, target_normals, voxel_size, max_distance):
"""
计算每个体素内的点云数据与目标点云数据的匹配度
:param source_points: 源点云数据
:param target_points: 目标点云数据
:param source_normals: 源点云法向量
:param target_normals: 目标点云法向量
:param voxel_size: 体素大小
:param max_distance: 最大匹配距离
:return: 匹配点对
"""
source_voxel_points, source_voxel_point_idx = voxel_downsample(source_points, voxel_size)
target_voxel_points, target_voxel_point_idx = voxel_downsample(target_points, voxel_size)
source_voxel_normals = np.zeros_like(source_voxel_points)
target_voxel_normals = np.zeros_like(target_voxel_points)
for i in range(len(source_voxel_point_idx)):
idx = source_voxel_point_idx[i]
source_voxel_normals[i] = np.mean(source_normals[idx], axis=0)
for i in range(len(target_voxel_point_idx)):
idx = target_voxel_point_idx[i]
target_voxel_normals[i] = np.mean(target_normals[idx], axis=0)
tree = KDTree(target_voxel_points)
dist, idx = tree.query(source_voxel_points, k=1, distance_upper_bound=max_distance)
valid_idx = np.where(np.isfinite(dist))[0]
source_voxel_points = source_voxel_points[valid_idx]
source_voxel_normals = source_voxel_normals[valid_idx]
target_voxel_points = target_voxel_points[idx[valid_idx]]
target_voxel_normals = target_voxel_normals[idx[valid_idx]]
source_voxel_features = np.hstack((source_voxel_points, source_voxel_normals))
target_voxel_features = np.hstack((target_voxel_points, target_voxel_normals))
tree = KDTree(target_voxel_features)
dist, idx = tree.query(source_voxel_features, k=1)
valid_idx = np.where(dist < max_distance)[0]
source_voxel_points = source_voxel_points[valid_idx]
target_voxel_points = target_voxel_points[idx[valid_idx]]
return source_voxel_points, target_voxel_points
```
4.根据匹配度进行点云配准:
```
from scipy.spatial.transform import Rotation
def icp(source_points, target_points, source_normals, target_normals, voxel_size, max_distance, max_iter=20):
"""
点云配准
:param source_points: 源点云数据
:param target_points: 目标点云数据
:param source_normals: 源点云法向量
:param target_normals: 目标点云法向量
:param voxel_size: 体素大小
:param max_distance: 最大匹配距离
:param max_iter: 最大迭代次数
:return: 配准后的源点云数据
"""
source_points = source_points.copy()
source_normals = source_normals.copy()
for i in range(max_iter):
source_voxel_points, target_voxel_points = compute_correspondences(source_points, target_points, source_normals,
target_normals, voxel_size, max_distance)
R, t = align_points(source_voxel_points, target_voxel_points)
source_points = source_points.dot(R.T) + t
source_normals = source_normals.dot(R.T)
return source_points
def align_points(source_points, target_points):
"""
计算点云配准的旋转矩阵和平移向量
:param source_points: 源点云数据
:param target_points: 目标点云数据
:return: 旋转矩阵和平移向量
"""
mu_s = np.mean(source_points, axis=0)
mu_t = np.mean(target_points, axis=0)
source_points_centered = source_points - mu_s
target_points_centered = target_points - mu_t
W = source_points_centered.T.dot(target_points_centered)
U, _, Vt = np.linalg.svd(W)
S = np.eye(3)
if np.linalg.det(U) * np.linalg.det(Vt) < 0:
S[2, 2] = -1
R = U.dot(S).dot(Vt)
t = mu_t - mu_s.dot(R.T)
return R, t
```
希望这些代码能够帮助到您。
阅读全文
相关推荐
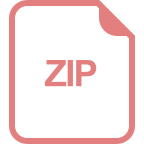
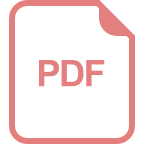
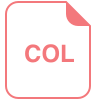
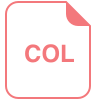
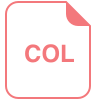
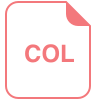
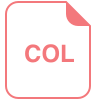
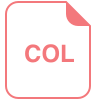
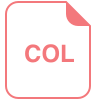
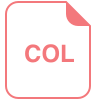
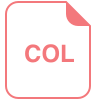
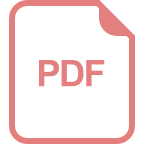
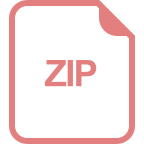
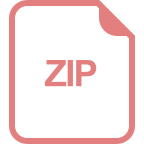
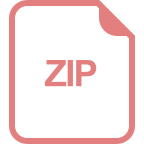