用Java写x(t)=-2tsin(t2),0≤t≤8,从0开始,以△t=0.01、0.02、0.04、0.08、0.1、0.25、0.5、1等为间隔,分别绘制不同△t下的x(t)的离散曲线
时间: 2024-04-30 19:17:22 浏览: 71
好的,您可以使用以下的Java代码来绘制不同△t下的x(t)的离散曲线:
```
import javax.swing.*;
import java.awt.*;
public class DiscretePlot extends JPanel {
private double[] xValues; // 存储x值的数组
private double[] tValues; // 存储t值的数组
private double deltaT; // △t的值
private int nPoints; // 计算出的点数
private int sampleRate; // 绘图的采样率
public DiscretePlot(double deltaT, int sampleRate) {
this.deltaT = deltaT;
this.nPoints = (int) Math.ceil(8 / deltaT); // 计算出的点数
this.xValues = new double[nPoints];
this.tValues = new double[nPoints];
this.sampleRate = sampleRate;
// 计算x(t)和t的离散值
for (int i = 0; i < nPoints; i++) {
tValues[i] = i * deltaT;
xValues[i] = -2 * tValues[i] * Math.sin(tValues[i] * tValues[i]);
}
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
// 设置画笔
Graphics2D g2d = (Graphics2D) g;
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setColor(Color.BLACK);
g2d.setStroke(new BasicStroke(2));
// 绘制x(t)的离散曲线
for (int i = 0; i < nPoints - 1; i++) {
int x1 = (int) Math.round(tValues[i] * sampleRate);
int y1 = (int) Math.round(xValues[i] * sampleRate);
int x2 = (int) Math.round(tValues[i + 1] * sampleRate);
int y2 = (int) Math.round(xValues[i + 1] * sampleRate);
g2d.drawLine(x1, getHeight() - y1, x2, getHeight() - y2); // 使用getHeight() - y是为了将坐标系上移,使其原点在左下角
}
}
public static void main(String[] args) {
JFrame f = new JFrame();
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setSize(800, 600);
JPanel p = new JPanel(new GridLayout(1, 0));
p.add(new DiscretePlot(0.01, 40));
p.add(new DiscretePlot(0.02, 20));
p.add(new DiscretePlot(0.04, 10));
p.add(new DiscretePlot(0.08, 5));
p.add(new DiscretePlot(0.1, 4));
p.add(new DiscretePlot(0.25, 2));
p.add(new DiscretePlot(0.5, 1));
p.add(new DiscretePlot(1, 1));
f.add(p);
f.setVisible(true);
}
}
```
在上述代码中,我们定义了一个`DiscretePlot`类,其构造方法需要传入△t的值和绘图的采样率。在构造方法中,我们计算出了x(t)和t的离散值,并保存在数组`xValues`和`tValues`中。在`paintComponent`方法中,我们使用Graphics2D的`drawLine`方法绘制了离散曲线。最后,在main方法中,我们创建了多个`DiscretePlot`实例,并添加到了一个JPanel中,然后将这个JPanel添加到了JFrame中。
您可以自行运行上述代码,然后观察不同△t下的x(t)的离散曲线。
相关推荐
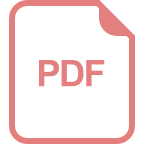
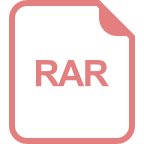














