使用thinkphp6和querylist写一个可缓存的网页代理工具,需要后续不同session每个页面继承主域名cookies,并且将页面的静态文件也做缓存,比如css、js、jpg
时间: 2024-06-12 09:04:58 浏览: 129
等文件。
思路:
1. 使用thinkphp6框架的HttpClient模块,发送请求获取网页内容。
2. 使用QueryList模块解析网页内容,提取需要的信息。
3. 将网页内容和静态文件缓存到本地,使用文件名作为缓存key。
4. 每次请求时,先从缓存中获取数据,如果缓存中没有,则重新发送请求并进行缓存。
5. 利用session共享机制,将主域名的cookies保存到session中,在每次请求时,将cookies添加到请求头中。
代码实现:
1. 在config目录下新建cache.php文件,配置缓存信息和缓存目录。
```php
<?php
return [
// 默认缓存驱动
'default' => env('cache.driver', 'file'),
// 缓存连接参数
'stores' => [
// 文件缓存
'file' => [
'type' => 'File',
'path' => app()->getRuntimePath() . 'cache',
'expire' => 0,
],
],
];
```
2. 在app目录下新建proxy目录,创建Proxy控制器。
```php
<?php
namespace app\proxy\controller;
use think\facade\Cache;
use think\facade\Session;
use think\facade\HttpClient;
use QL\QueryList;
class Proxy
{
// 缓存时间,单位秒
private $cacheTime = 3600;
public function index($url)
{
// 从缓存中获取网页内容和静态文件
$content = Cache::get($url);
if ($content) {
return $content;
}
// 获取主域名的cookies
$cookies = Session::get('cookies');
if (!$cookies) {
$response = HttpClient::get($url);
$cookies = $response->getCookies();
Session::set('cookies', $cookies);
}
// 发送请求获取网页内容
$response = HttpClient::get($url, [], [
'cookies' => $cookies,
'headers' => [
'User-Agent' => 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3',
],
]);
$html = $response->getBody();
// 解析网页内容,提取需要的信息
$ql = QueryList::html($html);
$ql->find('img')->map(function($item) {
$src = $item->src;
if ($src && strpos($src, 'http') === false) {
$item->src = $this->convertUrl($src);
}
});
$ql->find('link')->map(function($item) {
$href = $item->href;
if ($href && strpos($href, 'http') === false) {
$item->href = $this->convertUrl($href);
}
});
$ql->find('script')->map(function($item) {
$src = $item->src;
if ($src && strpos($src, 'http') === false) {
$item->src = $this->convertUrl($src);
}
});
$html = $ql->getHtml();
// 缓存网页内容和静态文件
Cache::set($url, $html, $this->cacheTime);
return $html;
}
// 将相对路径转换为绝对路径
private function convertUrl($url)
{
$base = $_SERVER['REQUEST_SCHEME'] . '://' . $_SERVER['HTTP_HOST'];
return $base . '/' . ltrim($url, '/');
}
}
```
3. 在路由中添加代理路由。
```php
use think\facade\Route;
Route::get('proxy/:url', 'proxy/Proxy/index');
```
使用方法:
访问http://localhost/proxy/www.baidu.com,即可代理访问百度网站,并缓存网页内容和静态文件。
阅读全文
相关推荐
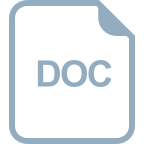
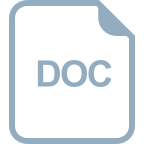
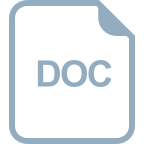
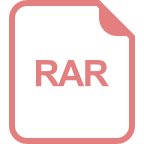
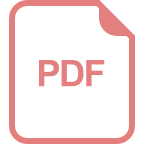
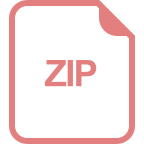
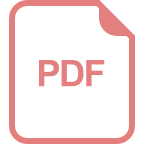
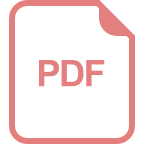
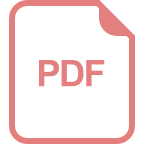
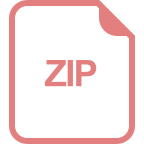
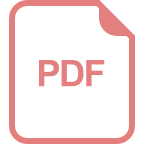
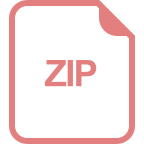
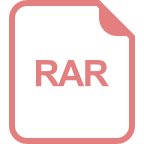
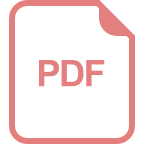
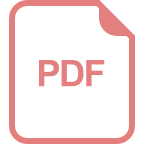
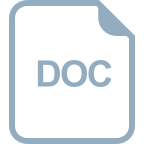
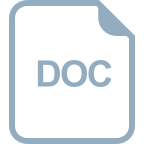
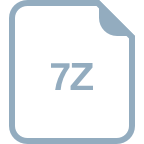