java 实现SFTP协议多个文件的上传
时间: 2023-10-27 22:04:53 浏览: 145
你可以使用 JSch 库来实现 SFTP 协议的多个文件上传。下面是一个简单的示例代码:
```java
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
import com.jcraft.jsch.SftpException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.Vector;
public class SftpUploader {
private String host;
private int port;
private String username;
private String password;
private String remoteDir;
public SftpUploader(String host, int port, String username, String password, String remoteDir) {
this.host = host;
this.port = port;
this.username = username;
this.password = password;
this.remoteDir = remoteDir;
}
public void uploadFiles(Vector<File> files) throws Exception {
JSch jsch = new JSch();
Session session = jsch.getSession(username, host, port);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelSftp channelSftp = (ChannelSftp) session.openChannel("sftp");
channelSftp.connect();
channelSftp.cd(remoteDir);
for (File file : files) {
try {
FileInputStream inputStream = new FileInputStream(file);
channelSftp.put(inputStream, file.getName());
inputStream.close();
} catch (FileNotFoundException e) {
System.err.println("File not found: " + file.getAbsolutePath());
} catch (SftpException e) {
System.err.println("Error uploading file: " + file.getAbsolutePath());
}
}
channelSftp.disconnect();
session.disconnect();
}
public static void main(String[] args) throws Exception {
String host = "example.com";
int port = 22;
String username = "username";
String password = "password";
String remoteDir = "/path/to/remote/directory";
Vector<File> files = new Vector<>();
files.add(new File("/path/to/local/file1"));
files.add(new File("/path/to/local/file2"));
SftpUploader uploader = new SftpUploader(host, port, username, password, remoteDir);
uploader.uploadFiles(files);
}
}
```
在上面的示例代码中,我们使用了 JSch 库来连接到 SFTP 服务器,并使用 ChannelSftp 类来执行 SFTP 操作。我们将要上传的文件列表存储在一个 Vector<File> 对象中,然后遍历该列表,将每个文件上传到远程服务器的指定目录中。
请注意,上面的代码仅作为示例,需要根据实际情况进行修改。例如,你需要替换 host、port、username、password 和 remoteDir 变量的值,以及将 files 列表替换为实际要上传的文件列表。
阅读全文
相关推荐












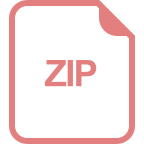





