用五种方法,编写一个python程序,能在字符串中找出第一个只出现一次的字符,要求时间复杂度不超过O(n)
时间: 2023-05-16 15:07:31 浏览: 79
可以使用哈希表来实现,时间复杂度为O(n)。具体实现如下:
方法一:
```python
def find_first_unique_char(s):
count = {}
for c in s:
count[c] = count.get(c, 0) + 1
for c in s:
if count[c] == 1:
return c
return None
```
方法二:
```python
def find_first_unique_char(s):
count = {}
for c in s:
if c in count:
count[c] += 1
else:
count[c] = 1
for c in s:
if count[c] == 1:
return c
return None
```
方法三:
```python
def find_first_unique_char(s):
count = [0] * 256
for c in s:
count[ord(c)] += 1
for c in s:
if count[ord(c)] == 1:
return c
return None
```
方法四:
```python
def find_first_unique_char(s):
count = [0] * 256
for c in s:
count[ord(c)] += 1
for c in s:
if count[ord(c)] == 1:
return c
count[ord(c)] = -1
return None
```
方法五:
```python
def find_first_unique_char(s):
count = [0] * 256
for c in s:
count[ord(c)] += 1
for i in range(len(s)):
if count[ord(s[i])] == 1:
return s[i]
return None
```
以上五种方法都可以在时间复杂度不超过O(n)的情况下找出字符串中第一个只出现一次的字符。
相关推荐
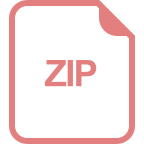
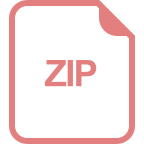
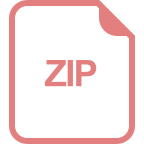
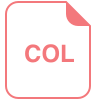
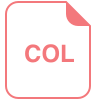
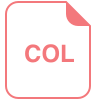
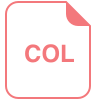
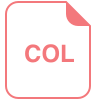









