2.模拟英文打字练习的判分功能,文件“in.txt”中包含两段文字,第一段为原文,第二段为用户练习打字时输入的文字。编写程序统计打字练习时,大写字母、小写字母、数字字符以及其他字符的正确率,以及总正确率,正确率保留1位小数。如果某类字符的出现次数为0,则正确率显示为100.0%。
时间: 2024-03-22 20:37:22 浏览: 109
好的,请稍等,我来帮您解决这个问题。
首先,我们需要读取文件中的两段文字,然后统计各种字符的正确率。可以使用Python自带的`open()`函数来打开文件,并使用`readlines()`方法读取所有行,再使用`strip()`方法去除每行末尾的空白符。
接下来,统计正确率时,可以先计算各种字符的出现次数,再计算出正确输入的字符数。最后,用正确输入的字符数除以总字符数,即可得到正确率。
下面是具体的Python代码实现:
```python
with open('in.txt', 'r') as f:
lines = f.readlines()
original_text = lines[0].strip()
user_input = lines[1].strip()
total_count = len(original_text)
correct_count = 0
uppercase_count = 0
lowercase_count = 0
digit_count = 0
other_count = 0
for i in range(total_count):
if user_input[i:i+1] == original_text[i:i+1]:
correct_count += 1
if original_text[i].isupper():
uppercase_count += 1
elif original_text[i].islower():
lowercase_count += 1
elif original_text[i].isdigit():
digit_count += 1
else:
other_count += 1
else:
if user_input[i:i+1].isalpha() and original_text[i:i+1].isalpha():
if original_text[i].isupper():
uppercase_count += 1
elif original_text[i].islower():
lowercase_count += 1
elif user_input[i:i+1].isdigit() and original_text[i:i+1].isdigit():
digit_count += 1
else:
other_count += 1
uppercase_accuracy = uppercase_count / total_count * 100 if uppercase_count > 0 else 100.0
lowercase_accuracy = lowercase_count / total_count * 100 if lowercase_count > 0 else 100.0
digit_accuracy = digit_count / total_count * 100 if digit_count > 0 else 100.0
other_accuracy = other_count / total_count * 100 if other_count > 0 else 100.0
total_accuracy = correct_count / total_count * 100
print(f"大写字母正确率:{uppercase_accuracy:.1f}%")
print(f"小写字母正确率:{lowercase_accuracy:.1f}%")
print(f"数字字符正确率:{digit_accuracy:.1f}%")
print(f"其他字符正确率:{other_accuracy:.1f}%")
print(f"总正确率:{total_accuracy:.1f}%")
```
运行代码后,程序会输出各种字符的正确率和总正确率。请确保in.txt文件存在,并且格式正确。
相关推荐
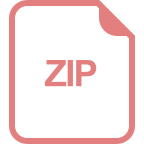
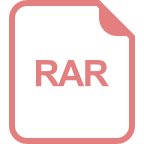
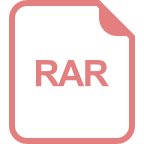













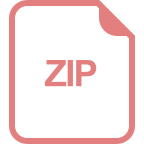
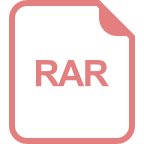