C++实现代码
时间: 2023-07-11 22:24:22 浏览: 54
以下是使用C++和OpenCV库实现图像标定和矫正的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取标定图像
Mat img = imread("calibration_image.png");
// 设置棋盘格内角点数目
int num_corners_x = 9;
int num_corners_y = 6;
// 生成棋盘格内角点坐标
vector<Point3f> obj;
for(int i = 0; i < num_corners_y; i++) {
for(int j = 0; j < num_corners_x; j++) {
obj.push_back(Point3f(j, i, 0));
}
}
// 寻找棋盘格内角点
vector<Point2f> corners;
bool found = findChessboardCorners(img, Size(num_corners_x, num_corners_y), corners);
// 如果找到了棋盘格内角点,进行标定和矫正操作
if (found) {
// 亚像素级别的角点精确化
cornerSubPix(img, corners, Size(11, 11), Size(-1, -1), TermCriteria(TermCriteria::EPS + TermCriteria::MAX_ITER, 30, 0.001));
// 计算相机矩阵和畸变系数
Mat cameraMatrix, distCoeffs;
vector<Mat> rvecs, tvecs;
vector<vector<Point3f>> objpoints(1, obj);
vector<vector<Point2f>> imgpoints(1, corners);
calibrateCamera(objpoints, imgpoints, img.size(), cameraMatrix, distCoeffs, rvecs, tvecs);
// 矫正图像
Mat undistorted;
Mat newCameraMatrix = getOptimalNewCameraMatrix(cameraMatrix, distCoeffs, img.size(), 1, img.size());
undistort(img, undistorted, cameraMatrix, distCoeffs, newCameraMatrix);
// 显示矫正前后的图像
imshow("Original Image", img);
imshow("Corrected Image", undistorted);
waitKey(0);
destroyAllWindows();
}
return 0;
}
```
在上面的代码中,我们首先读取标定图像,然后设置棋盘格内角点的数量,并生成角点坐标。接下来,我们使用OpenCV的 `findChessboardCorners` 函数寻找棋盘格内的角点,并对其进行亚像素级别的精确化处理。然后,我们使用 `calibrateCamera` 函数计算相机矩阵和畸变系数。最后,我们使用 `undistort` 函数对原始图像进行矫正,并使用 `getOptimalNewCameraMatrix` 函数计算新的相机矩阵和ROI区域。最后,我们展示原始图像和矫正后的图像。
请注意,这只是一个例子,实际应用中需要根据具体情况进行调整。
相关推荐
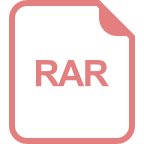















