为以下代码注释#include <iostream> using namespace std; class Date { public: Date(int month, int day, int year) { year = year; month = month; day = day; } Date(int month, int day) { year = 2000; month = month; day = day; } Date(); ~Date() {}; void show() const; private: int year{}; int month{}; int day{}; }; class People { private: int number, id; bool sex; Date birthday; public: People(int number, int id, bool sex, Date birthday); People(People &p); ~People() {}; void getNumber() const; void getSex() const; void getId() const; inline void setSex(bool sx) { sex = sx; }; void show(); }; void Date::show() const { cout << "生日:" << year << "年" << month << "月" << day << "日" << endl; } Date::Date() {} void People::show() { People::getSex(); People::getId(); People::getNumber(); People::birthday.show(); } void People::getId() const { cout << id << endl; } void People::getNumber() const { cout << number << endl; } void People::getSex() const { if (sex == 0) cout << "女" << endl; else cout << "男" << endl; } People::People(int number, int id, bool sex, Date birthday) { People::number = number; People::sex = sex; People::id = id; People::birthday = birthday; } People::People(People &p) { id = p.id; sex = p.sex; birthday = p.birthday; number = p.number; } int main() { Date dd(15, 2); People pp(456, 135132, 1, dd); pp.setSex(false); pp.show(); Date d(15, 2, 2001); People p(123, 130031, 1, d); p.show(); }
时间: 2024-01-03 10:06:01 浏览: 31
这是一个 C++ 的程序,实现了一个 People 类和一个 Date 类,其中 People 类有编号、身份证号、性别和生日等属性,以及显示个人信息的方法,Date 类有年、月、日等属性,以及显示日期信息的方法。程序的主函数中创建了两个 People 对象,分别使用不同的构造函数来初始化对象的属性,并调用 show 方法来输出对象的信息。以下是代码的注释:
```cpp
#include <iostream> // 引入输入输出流库
using namespace std; // 使用命名空间 std
// 定义 Date 类
class Date {
public:
// 构造函数,以月、日、年为参数
Date(int month, int day, int year) {
year = year; // 构造函数的参数名称与成员变量名称相同,需要使用 this 指针来区分,否则参数会覆盖成员变量
month = month;
day = day;
}
// 构造函数,以月、日为参数,年默认为 2000
Date(int month, int day) {
year = 2000;
month = month; // 同上,需要使用 this 指针来区分
day = day;
}
Date(); // 默认构造函数
~Date() {}; // 析构函数
void show() const; // 显示日期信息的方法
private:
int year{}; // 年,初始化为 0
int month{}; // 月,初始化为 0
int day{}; // 日,初始化为 0
};
// 定义 People 类
class People {
private:
int number, id; // 编号和身份证号
bool sex; // 性别,0 表示女,1 表示男
Date birthday; // 生日
public:
// 构造函数,以编号、身份证号、性别和生日为参数
People(int number, int id, bool sex, Date birthday);
People(People &p); // 拷贝构造函数
~People() {}; // 析构函数
void getNumber() const; // 获取编号的方法
void getSex() const; // 获取性别的方法
void getId() const; // 获取身份证号的方法
inline void setSex(bool sx) { // 设置性别的方法,使用内联函数
sex = sx;
};
void show(); // 显示个人信息的方法
};
// 在类外定义 Date 类的 show 方法,使用 const 关键字表示该方法不会修改对象的状态
void Date::show() const {
cout << "生日:" << year << "年" << month << "月" << day << "日" << endl;
}
Date::Date() {} // 默认构造函数为空实现
// 在类外定义 People 类的 show 方法
void People::show() {
People::getSex(); // 调用 getSex 方法来获取性别
People::getId(); // 调用 getId 方法来获取身份证号
People::getNumber(); // 调用 getNumber 方法来获取编号
People::birthday.show(); // 调用 Date 类的 show 方法来显示生日信息
}
// 在类外定义 People 类的 getId 方法,使用 const 关键字表示该方法不会修改对象的状态
void People::getId() const {
cout << id << endl;
}
// 在类外定义 People 类的 getNumber 方法,使用 const 关键字表示该方法不会修改对象的状态
void People::getNumber() const {
cout << number << endl;
}
// 在类外定义 People 类的 getSex 方法,使用 const 关键字表示该方法不会修改对象的状态
void People::getSex() const {
if (sex == 0) cout << "女" << endl;
else cout << "男" << endl;
}
// 在类外定义 People 类的构造函数,使用成员初始化列表来初始化对象的属性
People::People(int number, int id, bool sex, Date birthday) : number(number), id(id), sex(sex), birthday(birthday) {}
// 在类外定义 People 类的拷贝构造函数
People::People(People &p) {
id = p.id;
sex = p.sex;
birthday = p.birthday;
number = p.number;
}
// 主函数
int main() {
Date dd(15, 2); // 创建 Date 类的对象 dd,调用第二个构造函数来初始化对象
People pp(456, 135132, 1, dd); // 创建 People 类的对象 pp,调用第一个构造函数来初始化对象
pp.setSex(false); // 调用 setSex 方法来设置性别为女
pp.show(); // 调用 show 方法来输出 pp 对象的信息
Date d(15, 2, 2001); // 创建 Date 类的对象 d,调用第一个构造函数来初始化对象
People p(123, 130031, 1, d); // 创建 People 类的对象 p,调用第一个构造函数来初始化对象
p.show(); // 调用 show 方法来输出 p 对象的信息
}
```
相关推荐
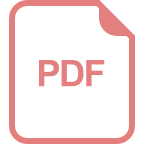
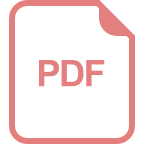
















