使用面向对象编程,自定义数组类,实现实现数组中数字之间的四则运算、内积运算和成员测试等功能
时间: 2024-11-17 10:29:37 浏览: 20
在面向对象编程中,自定义数组类是一个常见的做法,可以让你更好地控制数据结构并添加特定于需求的功能。例如,你可以创建一个名为`CustomArray`的类,它包含一个数组作为内部存储,并提供如下功能:
1. **初始化**:构造函数用于创建指定大小的空数组或根据给定值填充数组。
```java
public class CustomArray<T> {
private T[] array;
public CustomArray(int size) {
this.array = (T[]) new Object[size];
}
}
```
2. **四则运算**:为数组元素添加加法、减法、乘法和除法操作。
```java
public T add(int index, T value) {
// 确保索引有效
if (index >= 0 && index < array.length) {
return array[index] + value; // 假设T支持+操作
} else {
throw new IndexOutOfBoundsException();
}
}
// 其他算术操作类似,只需替换相应的运算符
```
3. **内积运算**:如果数组元素代表向量,可以计算两个数组的点积(假设它们具有相同的长度)。
```java
public T dotProduct(CustomArray<T> other) {
if (array.length != other.array.length) {
throw new IllegalArgumentException("Arrays must have the same length for dot product.");
}
T result = array[0].multiply(other.array[0]);
for (int i = 1; i < array.length; i++) {
result += array[i].multiply(other.array[i]);
}
return result;
}
// 假设T有一个multiply方法
```
4. **成员测试**:检查数组是否包含某个特定的元素。
```java
public boolean contains(T element) {
for (T item : array) {
if (item.equals(element)) {
return true;
}
}
return false;
}
```
阅读全文
相关推荐










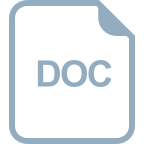






