c++ 实现发送http请求
时间: 2024-01-27 18:03:23 浏览: 42
以下是两种C++实现发送HTTP请求的例子:
1. 使用WinINet库发送HTTP请求
```cpp
#include <windows.h>
#include <wininet.h>
void urlopen(_TCHAR* url) {
HINTERNET hSession = InternetOpen(L"HTTP Example", INTERNET_OPEN_TYPE_DIRECT, NULL, NULL, 0);
if (hSession != NULL) {
HINTERNET hConnect = InternetOpenUrl(hSession, url, NULL, 0, INTERNET_FLAG_RELOAD, 0);
if (hConnect != NULL) {
char buffer[MAXSIZE];
DWORD bytesRead;
while (InternetReadFile(hConnect, buffer, sizeof(buffer), &bytesRead) && bytesRead > 0) {
// 处理接收到的数据
}
InternetCloseHandle(hConnect);
}
InternetCloseHandle(hSession);
}
}
int main() {
_TCHAR* url = L"http://coderzh.cnblogs.com";
urlopen(url);
return 0;
}
```
2. 使用WinHTTP库发送HTTP请求
```cpp
#include <windows.h>
#include <winhttp.h>
void urlopen(_TCHAR* url) {
HINTERNET hSession = WinHttpOpen(L"HTTP Example", WINHTTP_ACCESS_TYPE_DEFAULT_PROXY, WINHTTP_NO_PROXY_NAME, WINHTTP_NO_PROXY_BYPASS, 0);
if (hSession != NULL) {
HINTERNET hConnect = WinHttpOpenRequest(hSession, L"GET", url, NULL, WINHTTP_NO_REFERER, WINHTTP_DEFAULT_ACCEPT_TYPES, 0);
if (hConnect != NULL) {
if (WinHttpSendRequest(hConnect, WINHTTP_NO_ADDITIONAL_HEADERS, 0, WINHTTP_NO_REQUEST_DATA, 0, 0, 0)) {
if (WinHttpReceiveResponse(hConnect, NULL)) {
char buffer[MAXSIZE];
DWORD bytesRead;
while (WinInternetReadFile(hConnect, buffer, sizeof(buffer), &bytesRead) && bytesRead > 0) {
// 处理接收到的数据
}
}
}
WinHttpCloseHandle(hConnect);
}
WinHttpCloseHandle(hSession);
}
}
int main() {
_TCHAR* url = L"http://coderzh.cnblogs.com";
urlopen(url);
return 0;
}
```
相关推荐
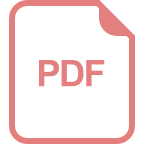
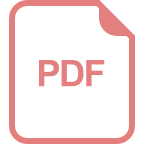
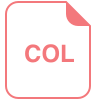
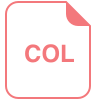
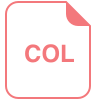











