将excel模板转化为html到前端页面填写
时间: 2023-08-14 19:02:44 浏览: 56
要将Excel模板转换为HTML,可以使用Java中的Apache POI库。以下是一个简单的示例代码,它将Excel模板转换为HTML:
```
import java.io.*;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.ss.util.CellAddress;
import org.apache.poi.ss.util.CellRangeAddress;
public class ExcelToHtmlConverter {
public static void main(String[] args) {
try {
// Load Excel workbook
FileInputStream inputStream = new FileInputStream(new File("path/to/excel/file"));
Workbook workbook = new XSSFWorkbook(inputStream);
// Get the first sheet
Sheet sheet = workbook.getSheetAt(0);
// Create a new HTML table
Writer output = new PrintWriter("path/to/html/file", "utf-8");
output.write("<table>");
// Loop through rows and columns to create HTML rows and cells
for (int i = sheet.getFirstRowNum(); i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
if (row != null) {
output.write("<tr>");
for (int j = row.getFirstCellNum(); j <= row.getLastCellNum(); j++) {
Cell cell = row.getCell(j);
if (cell != null) {
// Get cell value
String value = "";
switch (cell.getCellType()) {
case STRING:
value = cell.getStringCellValue();
break;
case NUMERIC:
value = Double.toString(cell.getNumericCellValue());
break;
case BOOLEAN:
value = Boolean.toString(cell.getBooleanCellValue());
break;
}
// Get cell address and span
CellAddress address = cell.getAddress();
int rowspan = 1, colspan = 1;
for (CellRangeAddress range : sheet.getMergedRegions()) {
if (range.isInRange(address)) {
rowspan = range.getLastRow() - range.getFirstRow() + 1;
colspan = range.getLastColumn() - range.getFirstColumn() + 1;
break;
}
}
// Create HTML cell
output.write("<td rowspan=\"" + rowspan + "\" colspan=\"" + colspan + "\">" + value + "</td>");
}
}
output.write("</tr>");
}
}
// Close the HTML table
output.write("</table>");
output.close();
// Close the workbook
workbook.close();
// Display success message
System.out.println("Excel converted to HTML successfully!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上代码将Excel模板转换为HTML文件并保存在指定的路径中。在前端页面中,可以使用任何HTML表格渲染库来呈现此表格。
相关推荐
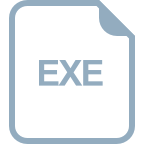
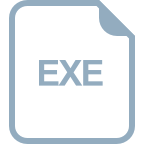














