java 多线程查询数据库
时间: 2023-10-12 16:00:35 浏览: 49
在 Java 中,你可以使用多线程来查询数据库。这样可以提高查询效率,特别是在需要处理大量数据的情况下。下面是一个简单的示例代码:
```java
import java.sql.*;
public class DatabaseQueryThread extends Thread {
private String query;
public DatabaseQueryThread(String query) {
this.query = query;
}
@Override
public void run() {
// 在这里执行数据库查询操作
try {
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(query);
// 处理查询结果
while (resultSet.next()) {
// ...
}
// 关闭连接
resultSet.close();
statement.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
// 创建多个查询线程
DatabaseQueryThread thread1 = new DatabaseQueryThread("SELECT * FROM table1");
DatabaseQueryThread thread2 = new DatabaseQueryThread("SELECT * FROM table2");
// 启动线程
thread1.start();
thread2.start();
// 等待线程执行完毕
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,我们创建了一个继承自 Thread 的自定义线程类 `DatabaseQueryThread`,并在 `run` 方法中执行数据库查询操作。我们可以创建多个 `DatabaseQueryThread` 实例,并分别传入不同的查询语句。然后,通过调用 `start` 方法启动线程,并使用 `join` 方法等待线程执行完毕。
需要注意的是,在实际的应用中,你可能需要使用连接池来管理数据库连接,以提高性能和资源利用率。此外,还需要注意线程安全性和并发控制,确保多个线程之间不会相互干扰或产生冲突。
相关推荐
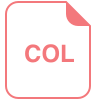
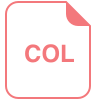
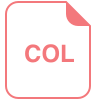
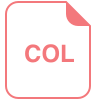
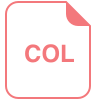








