java编写一个多线程的小应用程序,模拟小球碰壁反弹。
时间: 2024-12-10 16:48:46 浏览: 6
在Java中创建一个多线程小球碰撞反弹的应用程序通常涉及到Thread类或者更现代的ExecutorService和Runnable接口。这里是一个简单的示例,我们将创建一个BallThread类作为线程,它包含小球的位置信息和移动规则:
```java
public class BallThread extends Thread {
private int x = 0;
private int y = 0; // 小球初始位置
private int radius = 10; // 半径
private boolean isRunning = true;
public BallThread() {
start();
}
@Override
public void run() {
while (isRunning) {
// 模拟小球向四个方向移动
if (moveNorth() && hitsWall()) { // 如果撞到上边界
y -= radius;
} else if (moveSouth() && hitsWall()) { // 如果撞到底部
y += radius;
}
if (moveEast() && hitsWall()) { // 如果撞到右边
x += radius;
} else if (moveWest() && hitsWall()) { // 如果撞到左边
x -= radius;
}
// 更新屏幕显示
repaint();
try {
Thread.sleep(50); // 每隔50毫秒检查一次碰撞
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private boolean moveNorth() {
return ++y <= getHeight() - radius;
}
private boolean moveSouth() {
return y < radius;
}
private boolean moveEast() {
return ++x <= getWidth() - radius;
}
private boolean moveWest() {
return x < radius;
}
private boolean hitsWall() {
return Math.abs(x) >= radius || Math.abs(y) >= radius;
}
// 这些方法用于在Swing GUI框架中更新屏幕
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.fillOval(x, y, radius * 2, radius * 2); // 绘制小球
}
public void stopBall() {
isRunning = false;
}
}
// 主应用中启动球
public static void main(String[] args) {
JFrame frame = new JFrame("Ball Rebound");
BallThread ball = new BallThread();
frame.add(ball);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.setVisible(true);
}
```
阅读全文
相关推荐
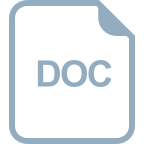
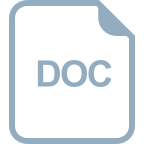
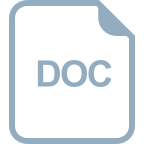
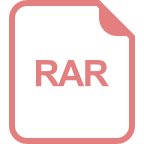
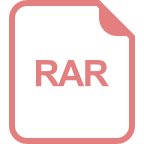
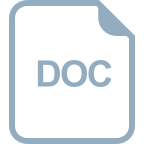
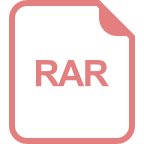
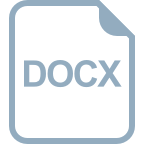
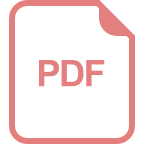
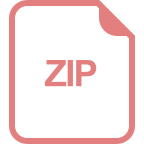
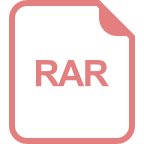
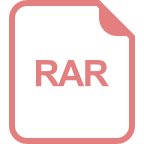
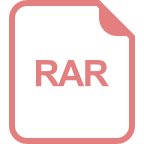
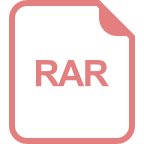