java 上传附件到/recourses/file目录下
时间: 2024-09-14 12:12:42 浏览: 39
在Java中,上传附件到服务器的`/recourses/file`目录下通常涉及以下几个步骤:
1. 创建HTTP客户端:可以使用Java自带的`HttpURLConnection`类,或者更高级的第三方库如Apache HttpClient或OkHttp来创建HTTP请求。
2. 设置请求方法:如果是上传文件,通常需要设置请求方法为`POST`。
3. 设置请求头:需要设置`Content-Type`为`multipart/form-data`,如果是大文件上传可能需要设置分块上传的参数。
4. 构建请求体:对于`multipart/form-data`类型,需要构建多部分表单数据,其中包含文件内容及其他可能的表单字段。
5. 发送请求:将构建好的请求发送到服务器,等待服务器响应。
6. 处理响应:根据服务器返回的状态码判断上传是否成功,并进行相应处理。
以下是使用Apache HttpClient实现上传文件到服务器的一个简单示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class FileUploader {
public static void main(String[] args) throws Exception {
// 创建HttpClient实例
try (CloseableHttpClient client = HttpClients.createDefault()) {
// 创建HttpPost实例,并设置目标URL
HttpPost post = new HttpPost("http://yourserver.com/recourses/file/upload");
// 构建多部分表单数据
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addBinaryBody(
"file",
new File("/path/to/your/file.txt"),
ContentType.APPLICATION_OCTET_STREAM,
"file.txt"
);
// 添加其他表单字段,如果有的话
// builder.addTextBody("paramName", "paramValue");
HttpEntity multipart = builder.build();
post.setEntity(multipart);
// 执行请求
CloseableHttpResponse response = client.execute(post);
// 检查响应状态
if (response.getStatusLine().getStatusCode() == 200) {
// 读取响应内容
String responseString = EntityUtils.toString(response.getEntity());
System.out.println("Upload successful: " + responseString);
} else {
System.out.println("Upload failed.");
}
// 关闭连接
response.close();
}
}
}
```
阅读全文
相关推荐
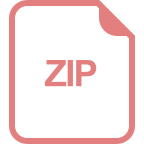
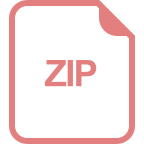
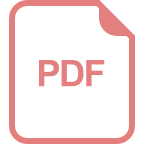

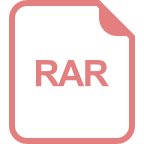
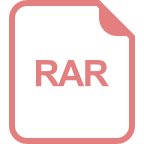
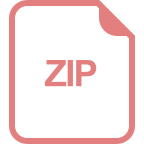
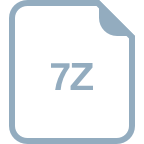
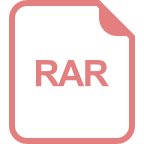
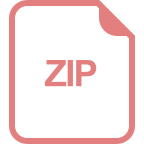
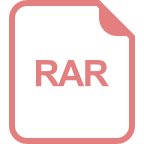
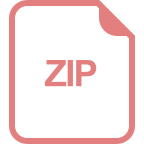