通过python给mysql中添加和读取图片
时间: 2024-02-25 08:58:22 浏览: 30
可以使用Python的MySQL驱动程序(如mysql-connector-python)和Python的Pillow库来实现将图片存储到MySQL数据库中并从数据库中读取图片。
以下是一个简单的示例代码,假设已经创建了名为"images"的数据库和名为"image_table"的表,其中包含"id" (int)和"image" (blob)两个字段:
```python
import mysql.connector
from mysql.connector import Error
from PIL import Image
# 将图像插入到MySQL数据库中
def write_file(filename):
try:
# 连接到MySQL数据库
conn = mysql.connector.connect(host='localhost',
database='images',
user='your_username',
password='your_password')
if conn.is_connected():
print('连接到MySQL数据库成功')
cursor = conn.cursor()
# 读取图像文件并将其转换为二进制数据
with open(filename, 'rb') as f:
img_data = f.read()
# 将图像数据插入到数据库中
insert_query = """INSERT INTO image_table
(id, image)
VALUES
(%s, %s)"""
record = (1, img_data)
cursor.execute(insert_query, record)
conn.commit()
print('图像已成功插入到数据库中')
except Error as e:
print(f'插入图像到数据库时发生错误:{e}')
finally:
cursor.close()
conn.close()
print('MySQL数据库连接已关闭')
# 从MySQL数据库中读取图像
def read_file():
try:
# 连接到MySQL数据库
conn = mysql.connector.connect(host='localhost',
database='images',
user='your_username',
password='your_password')
if conn.is_connected():
print('连接到MySQL数据库成功')
cursor = conn.cursor()
# 从数据库中获取图像数据
query = """SELECT image
FROM image_table
WHERE id = %s"""
record = (1,)
cursor.execute(query, record)
result = cursor.fetchone()
# 将图像数据转换为Image对象并保存为文件
with open('image.jpg', 'wb') as f:
f.write(result[0])
img = Image.open('image.jpg')
img.show()
except Error as e:
print(f'从数据库中读取图像时发生错误:{e}')
finally:
cursor.close()
conn.close()
print('MySQL数据库连接已关闭')
# 将图像插入到数据库中
write_file('image.jpg')
# 从数据库中读取图像并显示
read_file()
```
请注意,这只是一个简单的示例,实际使用时需要考虑更多的错误处理和优化。
相关推荐
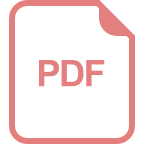
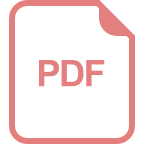
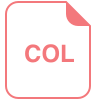
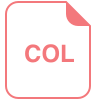
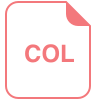
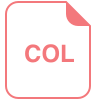
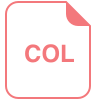









