用C++写一个宠物商店信息管理代码
时间: 2023-12-27 22:23:14 浏览: 69
以下是一个用C++写的宠物商店信息管理代码示例,实现了宠物信息的添加、删除、查询和修改功能:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Pet {
public:
string name; // 名称
string species; // 种类
int age; // 年龄
double price; // 价格
Pet(string name, string species, int age, double price) {
this->name = name;
this->species = species;
this->age = age;
this->price = price;
}
};
class PetShop {
private:
vector<Pet> pets;
public:
void add_pet(Pet pet) {
pets.push_back(pet);
cout << "宠物添加成功!" << endl;
}
void remove_pet(Pet pet) {
for (auto it = pets.begin(); it != pets.end(); ++it) {
if (it->name == pet.name && it->species == pet.species) {
pets.erase(it);
cout << "宠物删除成功!" << endl;
return;
}
}
cout << "宠物不存在!" << endl;
}
void search_pet(string name) {
for (auto pet : pets) {
if (pet.name == name) {
cout << "宠物已找到!" << endl;
cout << "名称:" << pet.name << ",种类:" << pet.species << ",年龄:" << pet.age << ",价格:" << pet.price << endl;
return;
}
}
cout << "宠物不存在!" << endl;
}
void modify_pet(Pet pet, string name="", string species="", int age=-1, double price=-1) {
for (auto& p : pets) {
if (p.name == pet.name && p.species == pet.species) {
if (!name.empty()) p.name = name;
if (!species.empty()) p.species = species;
if (age != -1) p.age = age;
if (price != -1) p.price = price;
cout << "宠物信息修改成功!" << endl;
return;
}
}
cout << "宠物不存在!" << endl;
}
};
int main() {
PetShop pet_shop;
Pet pet1("小黄", "狗", 2, 100);
pet_shop.add_pet(pet1);
pet_shop.search_pet("小黄");
pet_shop.modify_pet(pet1, "", "", 3, -1);
pet_shop.remove_pet(pet1);
return 0;
}
```
在上述代码中,`Pet` 类表示一个宠物对象,包含宠物的名称、种类、年龄和价格等信息。`PetShop` 类表示宠物商店,包含一个宠物列表,提供添加、删除、查询和修改宠物信息的方法。在测试代码中,首先创建一个宠物商店对象 `pet_shop`,并创建一个宠物对象 `pet1`,然后调用 `PetShop` 类的方法完成宠物信息的添加、查询、修改和删除操作。
阅读全文
相关推荐








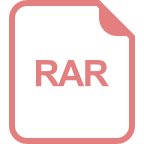






