帮我用yacc实现三地址代码生成器
时间: 2023-07-27 19:12:56 浏览: 56
好的,下面是一个简单的示例:
```c
%{
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int yylex();
void yyerror(const char *s);
int reg_count = 0; // 寄存器计数器
%}
%union {
char id[10];
int num;
}
%token <id> ID
%token <num> NUM
%token EQ PLUS MINUS TIMES DIV LPAREN RPAREN SEMICOLON
%left PLUS MINUS
%left TIMES DIV
%right UMINUS
%%
stmt_list:
stmt SEMICOLON
| stmt_list stmt SEMICOLON
;
stmt:
ID EQ expr {$$ = strdup($1);} {$$ = new_reg(); printf("%s = %s\n", $$, $3);}
;
expr:
expr PLUS expr {$$ = new_reg(); printf("%s = %s + %s\n", $$, $1, $3);}
| expr MINUS expr {$$ = new_reg(); printf("%s = %s - %s\n", $$, $1, $3);}
| expr TIMES expr {$$ = new_reg(); printf("%s = %s * %s\n", $$, $1, $3);}
| expr DIV expr {$$ = new_reg(); printf("%s = %s / %s\n", $$, $1, $3);}
| LPAREN expr RPAREN
| MINUS expr %prec UMINUS {$$ = new_reg(); printf("%s = -%s\n", $$, $2);}
| ID {$$ = strdup($1);}
| NUM {$$ = strdup($1);}
;
%%
int main() {
yyparse();
return 0;
}
int new_reg() {
char reg[10];
sprintf(reg, "t%d", ++reg_count);
return strdup(reg);
}
void yyerror(const char *s) {
fprintf(stderr, "%s\n", s);
}
int yylex() {
int c = getchar();
if (isdigit(c)) {
ungetc(c, stdin);
scanf("%d", &yylval.num);
return NUM;
}
else if (isalpha(c)) {
char *p = yylval.id;
do {
*p++ = c;
c = getchar();
} while (isalnum(c));
ungetc(c, stdin);
*p = '\0';
return ID;
}
else if (c == '+' || c == '-' || c == '*' || c == '/' || c == '(' || c == ')' || c == '=') {
return c;
}
else if (c == ';') {
return SEMICOLON;
}
else if (c == EOF) {
return 0;
}
else {
yyerror("Invalid character");
return 0;
}
}
```
这段代码实现了一个简单的表达式求值器,可以生成类似于以下格式的三地址代码:
```
t1 = 2 + 3
t2 = t1 - 4
t3 = t2 * 5
t4 = t3 / 6
result = t4
```
你可以根据需要修改代码以适应你的需求。
相关推荐
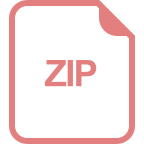
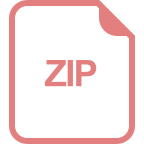
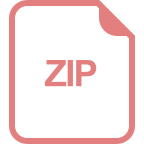














