利用java实现登陆页面
时间: 2023-11-17 08:04:22 浏览: 79
这里是一个简单的 Java 登录页面实现示例:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Login extends JFrame implements ActionListener {
private JLabel lblUsername, lblPassword;
private JTextField txtUsername;
private JPasswordField txtPassword;
private JButton btnLogin, btnCancel;
public Login() {
super("登录");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new GridBagLayout());
GridBagConstraints cs = new GridBagConstraints();
cs.fill = GridBagConstraints.HORIZONTAL;
lblUsername = new JLabel("用户名: ");
cs.gridx = 0;
cs.gridy = 0;
cs.gridwidth = 1;
panel.add(lblUsername, cs);
txtUsername = new JTextField(20);
cs.gridx = 1;
cs.gridy = 0;
cs.gridwidth = 2;
panel.add(txtUsername, cs);
lblPassword = new JLabel("密码: ");
cs.gridx = 0;
cs.gridy = 1;
cs.gridwidth = 1;
panel.add(lblPassword, cs);
txtPassword = new JPasswordField(20);
cs.gridx = 1;
cs.gridy = 1;
cs.gridwidth = 2;
panel.add(txtPassword, cs);
panel.setBorder(BorderFactory.createLineBorder(Color.GRAY));
btnLogin = new JButton("登录");
btnLogin.addActionListener(this);
btnCancel = new JButton("取消");
btnCancel.addActionListener(this);
JPanel bp = new JPanel();
bp.add(btnLogin);
bp.add(btnCancel);
getContentPane().add(panel, BorderLayout.CENTER);
getContentPane().add(bp, BorderLayout.PAGE_END);
pack();
setLocationRelativeTo(null);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnCancel) {
dispose();
} else if (e.getSource() == btnLogin) {
String username = txtUsername.getText();
String password = new String(txtPassword.getPassword());
if (isValidLogin(username, password)) {
JOptionPane.showMessageDialog(Login.this, "登录成功!",
"登录", JOptionPane.INFORMATION_MESSAGE);
} else {
JOptionPane.showMessageDialog(Login.this,
"用户名或密码不正确,请重试!", "登录",
JOptionPane.ERROR_MESSAGE);
}
}
}
private boolean isValidLogin(String username, String password) {
// 这里可以添加你的登录验证代码
// 返回 true 表示验证通过,返回 false 表示验证失败
return username.equals("admin") && password.equals("123456");
}
public static void main(String[] args) {
new Login().setVisible(true);
}
}
```
该示例使用了 Java Swing 库来创建界面组件,包括标签、文本框、密码框、按钮等。实现时,需要先定义各个组件的位置和大小,再将它们添加到容器中。对于按钮,需要添加 ActionListener 监听器,以便在用户点击按钮时触发相应的事件。
该示例中的登录验证只是一个简单的示例,实际开发中需要根据具体需求进行修改。如果需要与数据库交互进行验证,可以使用 JDBC 库来实现。
阅读全文
相关推荐
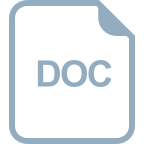
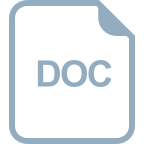
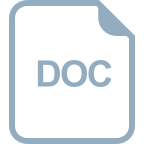
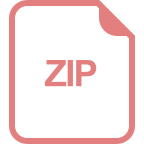
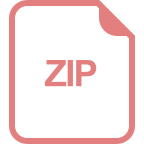
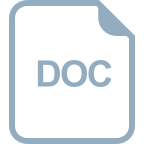
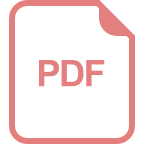
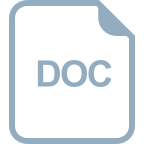
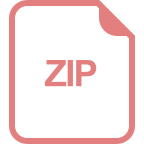
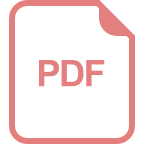
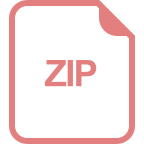
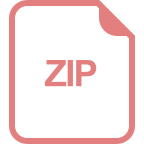
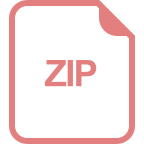
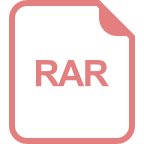
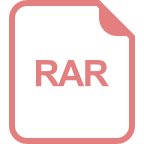
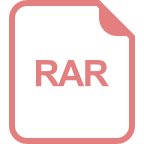
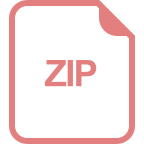
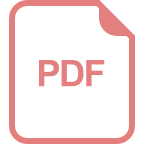
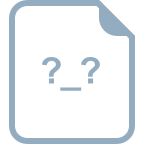