FFmpeg AVFrame转为int8_t *
时间: 2023-03-19 21:25:12 浏览: 163
In order to convert an AVFrame from FFmpeg to an int8_t* array, you can use the following code:
```c
#include <stdint.h>
// Function to convert AVFrame to int8_t*
int8_t* avframe_to_int8_array(AVFrame* frame) {
int width = frame->width;
int height = frame->height;
int size = width * height * 3; // assuming 3 channels (RGB)
int8_t* buffer = (int8_t*) malloc(size);
int index = 0;
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
buffer[index++] = frame->data[0][y * frame->linesize[0] + x * 3];
buffer[index++] = frame->data[0][y * frame->linesize[0] + x * 3 + 1];
buffer[index++] = frame->data[0][y * frame->linesize[0] + x * 3 + 2];
}
}
return buffer;
}
```
This function assumes that the input AVFrame has 3 channels (RGB). If the input frame has a different number of channels, you will need to modify the code accordingly.
Also, please note that the returned buffer is allocated dynamically using `malloc()`, so it is important to free the buffer when you are done using it:
```c
int8_t* buffer = avframe_to_int8_array(frame);
// use buffer...
free(buffer);
```
阅读全文
相关推荐
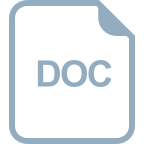
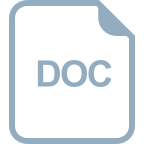
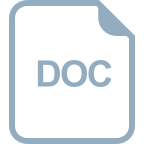




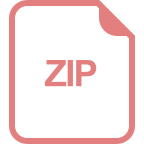
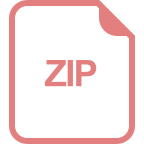
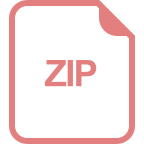
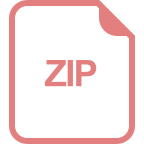
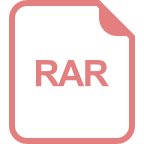
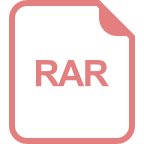
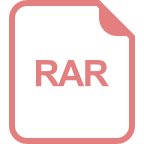
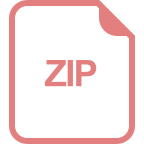
