c语言用while编译四则运算,如何使用c语言实现四则运算,详细思路很重要啊(越详细越好~),可以木有代码(急)...
时间: 2024-01-12 07:02:18 浏览: 75
实现四则运算的基本思路如下:
1. 读入表达式:从控制台或文件中读入待计算的表达式字符串,例如"3+4*2-1"。
2. 将表达式转换为逆波兰表达式:逆波兰表达式是一种将操作符放在操作数之后的表达式,例如"3 4 2 * + 1 -"。转换过程可以使用栈来实现,具体步骤如下:
a. 初始化两个栈:一个操作符栈和一个输出栈。
b. 从左到右扫描表达式字符串,如果是数字则直接压入输出栈,如果是操作符则判断其优先级与操作符栈顶元素的优先级,如果优先级低于或等于栈顶元素则弹出栈顶元素并压入输出栈,直到栈顶元素优先级小于该操作符,然后将该操作符压入操作符栈。
c. 扫描完表达式字符串后,将操作符栈中的所有元素弹出并压入输出栈。
d. 输出栈中的元素即为逆波兰表达式。
3. 计算逆波兰表达式:从左到右扫描逆波兰表达式,如果是数字则压入栈中,如果是操作符则弹出栈顶的两个元素进行相应的运算并将结果压入栈中,直到扫描完表达式为止。最后栈中剩下的元素即为计算结果。
下面是一个简单的示例代码(仅供参考,具体实现方式可能会有所不同):
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#define MAX_EXPR_LEN 100 // 表达式串的最大长度
#define MAX_STACK_SIZE MAX_EXPR_LEN // 栈的最大容量
typedef enum {
OP_PLUS,
OP_MINUS,
OP_MULTIPLY,
OP_DIVIDE
} Operator; // 操作符枚举类型
typedef struct {
int top;
int data[MAX_STACK_SIZE];
} Stack;
void stack_init(Stack *s) {
s->top = -1;
}
void stack_push(Stack *s, int val) {
if (s->top >= MAX_STACK_SIZE - 1) {
fprintf(stderr, "Stack overflow\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = val;
}
int stack_pop(Stack *s) {
if (s->top < 0) {
fprintf(stderr, "Stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
int stack_top(Stack *s) {
if (s->top < 0) {
fprintf(stderr, "Stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top];
}
int is_operator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
Operator operator_from_char(char ch) {
switch (ch) {
case '+': return OP_PLUS;
case '-': return OP_MINUS;
case '*': return OP_MULTIPLY;
case '/': return OP_DIVIDE;
default:
fprintf(stderr, "Invalid operator: %c\n", ch);
exit(EXIT_FAILURE);
}
}
int operator_priority(Operator op) {
switch (op) {
case OP_PLUS:
case OP_MINUS:
return 1;
case OP_MULTIPLY:
case OP_DIVIDE:
return 2;
default:
fprintf(stderr, "Invalid operator: %d\n", op);
exit(EXIT_FAILURE);
}
}
void convert_to_postfix(char *expr, char *postfix) {
Stack op_stack;
stack_init(&op_stack);
int len = strlen(expr);
int postfix_len = 0;
for (int i = 0; i < len; i++) {
char ch = expr[i];
if (isdigit(ch)) {
// 数字直接输出
postfix[postfix_len++] = ch;
while (isdigit(expr[i + 1])) {
postfix[postfix_len++] = expr[++i];
}
postfix[postfix_len++] = ' '; // 数字后面要加一个空格
} else if (is_operator(ch)) {
// 操作符入栈
Operator op = operator_from_char(ch);
while (op_stack.top >= 0 && operator_priority(op) <= operator_priority((Operator) stack_top(&op_stack))) {
postfix[postfix_len++] = stack_pop(&op_stack);
postfix[postfix_len++] = ' ';
}
stack_push(&op_stack, op);
} else if (ch == '(') {
// 左括号入栈
stack_push(&op_stack, ch);
} else if (ch == ')') {
// 右括号弹出栈中的操作符直到左括号
while (op_stack.top >= 0 && stack_top(&op_stack) != '(') {
postfix[postfix_len++] = stack_pop(&op_stack);
postfix[postfix_len++] = ' ';
}
if (op_stack.top < 0 || stack_pop(&op_stack) != '(') {
fprintf(stderr, "Mismatched parentheses\n");
exit(EXIT_FAILURE);
}
} else if (isspace(ch)) {
// 忽略空白字符
continue;
} else {
fprintf(stderr, "Invalid character: %c\n", ch);
exit(EXIT_FAILURE);
}
}
// 弹出剩余的操作符
while (op_stack.top >= 0) {
char op = stack_pop(&op_stack);
if (op == '(') {
fprintf(stderr, "Mismatched parentheses\n");
exit(EXIT_FAILURE);
}
postfix[postfix_len++] = op;
postfix[postfix_len++] = ' ';
}
postfix[postfix_len] = '\0';
}
int evaluate_postfix(char *postfix) {
Stack val_stack;
stack_init(&val_stack);
int len = strlen(postfix);
for (int i = 0; i < len; i++) {
char ch = postfix[i];
if (isdigit(ch)) {
// 数字入栈
int val = ch - '0';
while (isdigit(postfix[i + 1])) {
val = val * 10 + (postfix[++i] - '0');
}
stack_push(&val_stack, val);
} else if (is_operator(ch)) {
// 弹出栈顶的两个数字进行计算并将结果入栈
int val2 = stack_pop(&val_stack);
int val1 = stack_pop(&val_stack);
int result;
switch (operator_from_char(ch)) {
case OP_PLUS: result = val1 + val2; break;
case OP_MINUS: result = val1 - val2; break;
case OP_MULTIPLY: result = val1 * val2; break;
case OP_DIVIDE: result = val1 / val2; break;
}
stack_push(&val_stack, result);
} else if (isspace(ch)) {
// 忽略空白字符
continue;
} else {
fprintf(stderr, "Invalid character: %c\n", ch);
exit(EXIT_FAILURE);
}
}
if (val_stack.top != 0) {
fprintf(stderr, "Invalid expression\n");
exit(EXIT_FAILURE);
}
return stack_pop(&val_stack);
}
int main() {
char expr[MAX_EXPR_LEN];
printf("Enter an expression: ");
fgets(expr, MAX_EXPR_LEN, stdin);
char postfix[MAX_EXPR_LEN];
convert_to_postfix(expr, postfix);
printf("Postfix notation: %s\n", postfix);
int result = evaluate_postfix(postfix);
printf("Result: %d\n", result);
return 0;
}
```
阅读全文
相关推荐
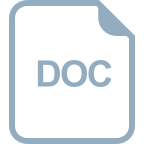
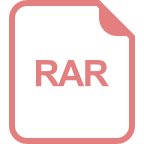
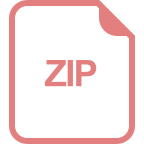
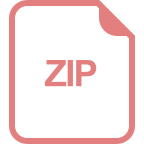
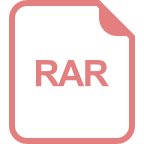
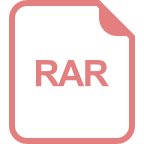
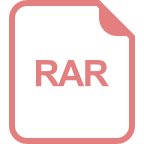
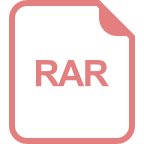
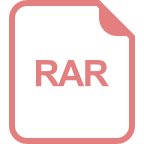
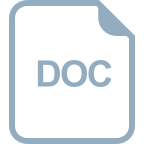
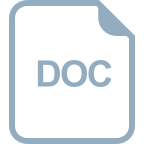
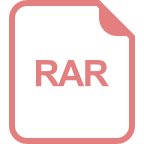
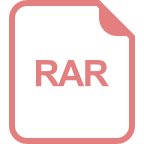
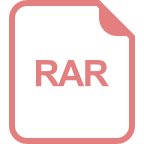
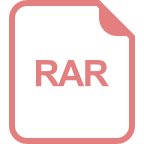
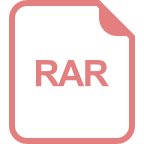