帮我用c语言的链表来写个歌曲管理系统,并保存到文件里,还可以对文件里的歌曲信息进行增删查改
时间: 2023-06-14 09:04:58 浏览: 104
好的,以下是一个简单的歌曲管理系统的代码示例,使用了链表来存储歌曲信息,并且可以将歌曲信息保存到文件中。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Song {
char title[50];
char artist[50];
char album[50];
int year;
struct Song *next;
} Song;
Song *head = NULL;
void insertSong(char *title, char *artist, char *album, int year) {
Song *newSong = (Song*) malloc(sizeof(Song));
strcpy(newSong->title, title);
strcpy(newSong->artist, artist);
strcpy(newSong->album, album);
newSong->year = year;
newSong->next = NULL;
if (head == NULL) {
head = newSong;
} else {
Song *current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newSong;
}
}
void displaySongs() {
if (head == NULL) {
printf("No songs\n");
} else {
Song *current = head;
while (current != NULL) {
printf("%s - %s (%s, %d)\n", current->title, current->artist, current->album, current->year);
current = current->next;
}
}
}
void saveToFile() {
FILE *fp = fopen("songs.txt", "w");
if (fp == NULL) {
printf("Error opening file\n");
} else {
Song *current = head;
while (current != NULL) {
fprintf(fp, "%s,%s,%s,%d\n", current->title, current->artist, current->album, current->year);
current = current->next;
}
fclose(fp);
printf("Saved to file\n");
}
}
void loadFromFile() {
FILE *fp = fopen("songs.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
} else {
char line[200];
while (fgets(line, sizeof(line), fp)) {
char *title = strtok(line, ",");
char *artist = strtok(NULL, ",");
char *album = strtok(NULL, ",");
char *yearStr = strtok(NULL, ",");
int year = atoi(yearStr);
insertSong(title, artist, album, year);
}
fclose(fp);
printf("Loaded from file\n");
}
}
void deleteSong(char *title) {
if (head == NULL) {
printf("No songs\n");
} else {
Song *current = head;
Song *previous = NULL;
while (current != NULL) {
if (strcmp(current->title, title) == 0) {
if (previous == NULL) {
head = current->next;
} else {
previous->next = current->next;
}
free(current);
printf("Deleted song: %s\n", title);
return;
}
previous = current;
current = current->next;
}
printf("Song not found: %s\n", title);
}
}
void editSong(char *title) {
if (head == NULL) {
printf("No songs\n");
} else {
Song *current = head;
while (current != NULL) {
if (strcmp(current->title, title) == 0) {
printf("Enter new title: ");
scanf("%s", current->title);
printf("Enter new artist: ");
scanf("%s", current->artist);
printf("Enter new album: ");
scanf("%s", current->album);
printf("Enter new year: ");
scanf("%d", ¤t->year);
printf("Song updated\n");
return;
}
current = current->next;
}
printf("Song not found: %s\n", title);
}
}
int main() {
loadFromFile();
int choice;
char title[50];
char artist[50];
char album[50];
int year;
do {
printf("1. Add song\n");
printf("2. Display songs\n");
printf("3. Save to file\n");
printf("4. Delete song\n");
printf("5. Edit song\n");
printf("6. Quit\n");
printf("Enter choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter title: ");
scanf("%s", title);
printf("Enter artist: ");
scanf("%s", artist);
printf("Enter album: ");
scanf("%s", album);
printf("Enter year: ");
scanf("%d", &year);
insertSong(title, artist, album, year);
break;
case 2:
displaySongs();
break;
case 3:
saveToFile();
break;
case 4:
printf("Enter title of song to delete: ");
scanf("%s", title);
deleteSong(title);
break;
case 5:
printf("Enter title of song to edit: ");
scanf("%s", title);
editSong(title);
break;
case 6:
break;
default:
printf("Invalid choice\n");
break;
}
} while (choice != 6);
return 0;
}
```
此代码使用了以下操作:
- `insertSong`:在链表中插入新的歌曲信息。
- `displaySongs`:显示链表中所有的歌曲信息。
- `saveToFile`:将链表中的歌曲信息保存到文件中。
- `loadFromFile`:从文件中加载歌曲信息,并将其插入链表中。
- `deleteSong`:删除链表中指定的歌曲信息。
- `editSong`:编辑链表中指定的歌曲信息。
你可以根据自己的需求修改这些操作。
相关推荐
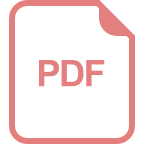














