帮我写一段文件映射内存读写文件的代码 linux平台上的
时间: 2023-08-28 17:16:41 浏览: 115
好的,以下是一个简单的在 Linux 平台上实现文件映射内存读写的代码示例:
```c++
#include <iostream>
#include <sys/mman.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <cstring>
using namespace std;
int main() {
// 打开文件
int fd = open("test.txt", O_RDWR | O_CREAT, S_IRUSR | S_IWUSR);
if (fd == -1) {
cout << "Failed to open file" << endl;
return 1;
}
// 获取文件大小
struct stat sb;
if (fstat(fd, &sb) == -1) {
cout << "Failed to get file size" << endl;
close(fd);
return 1;
}
// 创建文件映射
char* addr = (char*) mmap(NULL, sb.st_size, PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (addr == MAP_FAILED) {
cout << "Failed to create file mapping" << endl;
close(fd);
return 1;
}
// 读写文件内容
strcpy(addr, "Hello, World!");
cout << "Write data to file: " << addr << endl;
// 解除映射,关闭文件句柄
munmap(addr, sb.st_size);
close(fd);
return 0;
}
```
同样的,这段代码包括了打开文件、获取文件大小、创建文件映射、读写文件内容、解除映射、关闭文件句柄等步骤。其中,我们使用 `open` 函数打开文件,使用 `fstat` 函数获取文件大小,使用 `mmap` 函数创建文件映射。我们还使用了 `strcpy` 函数将字符串写入文件。需要注意的是,这里的 `addr` 是指向映射到内存的文件的指针,可以直接对它进行读写操作。最后,我们使用 `munmap` 函数解除映射,关闭文件句柄。
阅读全文
相关推荐
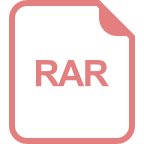
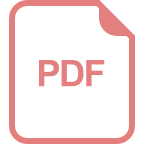
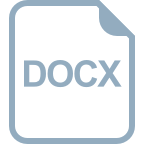
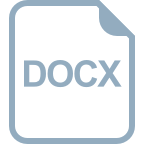
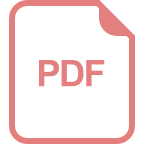
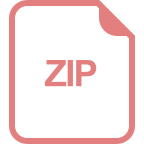
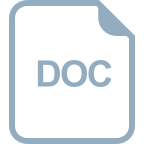
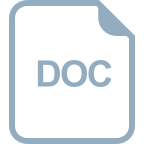
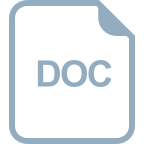
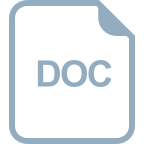
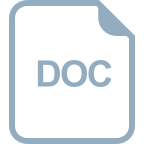
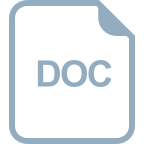
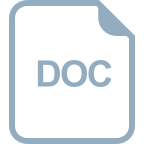
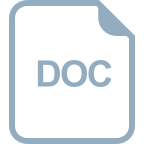
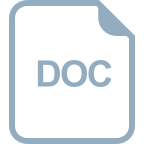
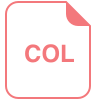