用html实现 日期 时间 到今年结束的倒计时三种功能,日期要有年月日和星期,要求时间和倒计时精确到秒。
时间: 2023-06-10 14:08:38 浏览: 100
以下是一个简单的实现:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>倒计时</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
}
h1 {
font-size: 48px;
margin-top: 50px;
}
p {
font-size: 24px;
margin-top: 20px;
}
</style>
</head>
<body>
<h1>倒计时</h1>
<p id="date"></p>
<p id="time"></p>
<p id="countdown"></p>
<script>
function updateDate() {
const now = new Date();
const year = now.getFullYear();
const month = now.getMonth() + 1;
const day = now.getDate();
const weekDays = ['日', '一', '二', '三', '四', '五', '六'];
const weekDay = weekDays[now.getDay()];
const dateStr = `${year}年${month}月${day}日 星期${weekDay}`;
document.getElementById('date').textContent = dateStr;
}
function updateTime() {
const now = new Date();
const hour = now.getHours();
const minute = now.getMinutes();
const second = now.getSeconds();
const timeStr = `${hour.toString().padStart(2, '0')}:${minute.toString().padStart(2, '0')}:${second.toString().padStart(2, '0')}`;
document.getElementById('time').textContent = timeStr;
}
function updateCountdown() {
const now = new Date();
const endOfYear = new Date(now.getFullYear() + 1, 0, 1);
const diff = endOfYear - now;
const days = Math.floor(diff / (1000 * 60 * 60 * 24));
const hours = Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((diff % (1000 * 60)) / 1000);
const countdownStr = `距离今年结束还剩${days}天${hours}小时${minutes}分钟${seconds}秒`;
document.getElementById('countdown').textContent = countdownStr;
}
updateDate();
updateTime();
updateCountdown();
setInterval(updateTime, 1000);
setInterval(updateCountdown, 1000);
</script>
</body>
</html>
```
在这个例子中,我们使用了 JavaScript 来更新日期、时间和倒计时。`updateDate` 函数获取当前日期,并将其格式化为年月日和星期的字符串。`updateTime` 函数获取当前时间并将其格式化为小时、分钟和秒的字符串。`updateCountdown` 函数计算距离今年结束还有多少时间,并将其格式化为字符串。
在页面加载时,我们调用这三个函数来初始化页面的内容。然后,我们使用 `setInterval` 函数来每秒钟更新一次时间和倒计时。
这只是一个简单的例子,可以根据需要进行修改和扩展。
阅读全文
相关推荐
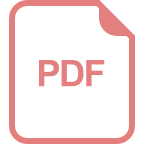
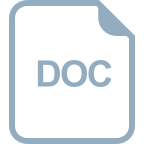
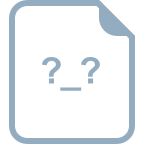
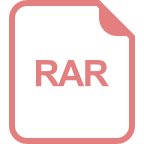
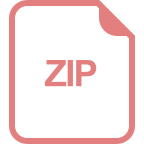
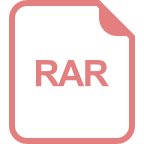
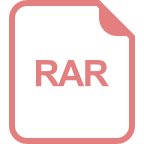
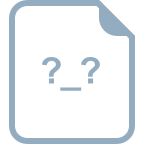
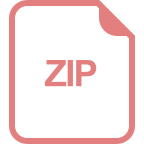
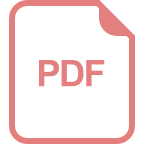
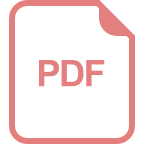
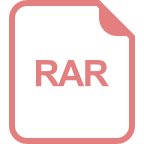
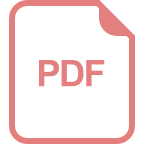
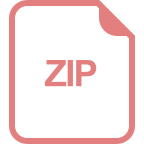
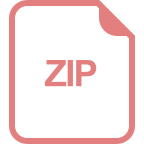
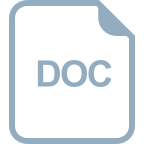