用c++人工智能学习 遗传算法实现图片再现源代码
时间: 2024-05-02 14:23:36 浏览: 100
遗传算法可以用于图像重建和图像生成的问题。以下是一些实现图像重建的遗传算法的源代码示例:
首先,需要将图像转换为一个二进制字符串表示。可以将每个像素点视为一个二进制数,然后将所有像素点组成的二进制序列表示整个图像。接下来,可以使用遗传算法对这个二进制序列进行优化,使得它能够重建出原始图像。
以下是一个使用遗传算法进行图像重建的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define POPSIZE 1000
#define ELITRATE 0.10
#define MUTATIONRATE 0.25
#define MAXGENERATIONS 1000
typedef struct {
char *gene; // 二进制串
double fitness; // 适应度
} Individual;
typedef struct {
Individual *individuals;
int size;
} Population;
char *target; // 目标图像的二进制串表示
int width, height; // 图像的宽度和高度
// 初始化种群
Population *initialize_population() {
Population *pop = (Population *) malloc(sizeof(Population));
pop->individuals = (Individual *) malloc(sizeof(Individual) * POPSIZE);
pop->size = POPSIZE;
for (int i = 0; i < POPSIZE; i++) {
pop->individuals[i].gene = (char *) malloc(sizeof(char) * strlen(target));
for (int j = 0; j < strlen(target); j++) {
if ((double) rand() / RAND_MAX < 0.5) {
pop->individuals[i].gene[j] = '0';
} else {
pop->individuals[i].gene[j] = '1';
}
}
}
return pop;
}
// 计算适应度
void calculate_fitness(Population *pop) {
for (int i = 0; i < pop->size; i++) {
double error = 0.0;
for (int j = 0; j < strlen(target); j++) {
if (pop->individuals[i].gene[j] != target[j]) {
error++;
}
}
pop->individuals[i].fitness = 1.0 / (error + 1.0);
}
}
// 选择优秀的个体
void select(Population *pop, Population *new_pop) {
int elitcount = (int) (ELITRATE * pop->size);
for (int i = 0; i < elitcount; i++) {
int elite_idx = 0;
double elite_fitness = 0.0;
for (int j = 0; j < pop->size; j++) {
if (pop->individuals[j].fitness > elite_fitness) {
elite_fitness = pop->individuals[j].fitness;
elite_idx = j;
}
}
new_pop->individuals[i] = pop->individuals[elite_idx];
pop->individuals[elite_idx].fitness = 0.0;
}
}
// 交叉操作
void crossover(Population *pop, Population *new_pop) {
int elitcount = (int) (ELITRATE * pop->size);
for (int i = elitcount; i < pop->size; i++) {
int idx1 = rand() % pop->size;
int idx2 = rand() % pop->size;
int spos = rand() % strlen(target);
new_pop->individuals[i].gene = (char *) malloc(sizeof(char) * strlen(target));
for (int j = 0; j < strlen(target); j++) {
if (j < spos) {
new_pop->individuals[i].gene[j] = pop->individuals[idx1].gene[j];
} else {
new_pop->individuals[i].gene[j] = pop->individuals[idx2].gene[j];
}
}
}
}
// 变异操作
void mutate(Population *pop) {
for (int i = (int) (ELITRATE * pop->size); i < pop->size; i++) {
for (int j = 0; j < strlen(target); j++) {
if ((double) rand() / RAND_MAX < MUTATIONRATE) {
if (pop->individuals[i].gene[j] == '0') {
pop->individuals[i].gene[j] = '1';
} else {
pop->individuals[i].gene[j] = '0';
}
}
}
}
}
// 找到最佳个体
Individual find_best_individual(Population *pop) {
Individual best = pop->individuals[0];
for (int i = 1; i < pop->size; i++) {
if (pop->individuals[i].fitness > best.fitness) {
best = pop->individuals[i];
}
}
return best;
}
int main(int argc, char *argv[]) {
srand((unsigned) time(NULL));
// 读取目标图像
FILE *fp;
fp = fopen("image.bin", "rb");
fread(&width, sizeof(int), 1, fp);
fread(&height, sizeof(int), 1, fp);
target = (char *) malloc(sizeof(char) * width * height * 8);
fread(target, sizeof(char), width * height * 8, fp);
fclose(fp);
// 初始化种群
Population *pop = initialize_population();
// 进化过程
for (int i = 0; i < MAXGENERATIONS; i++) {
Population *new_pop = (Population *) malloc(sizeof(Population));
new_pop->individuals = (Individual *) malloc(sizeof(Individual) * POPSIZE);
new_pop->size = POPSIZE;
// 计算适应度
calculate_fitness(pop);
// 选择优秀的个体
select(pop, new_pop);
// 交叉操作
crossover(pop, new_pop);
// 变异操作
mutate(new_pop);
// 更新种群
free(pop->individuals);
free(pop);
pop = new_pop;
// 输出最佳个体
Individual best = find_best_individual(pop);
printf("Generation %d: fitness = %f\n", i, best.fitness);
}
// 输出最终结果
Individual best = find_best_individual(pop);
printf("Best individual: fitness = %f\n", best.fitness);
for (int i = 0; i < strlen(target); i++) {
printf("%c", best.gene[i]);
}
printf("\n");
return 0;
}
```
以上代码仅作为参考,实际上需要根据具体的问题进行修改和优化。
阅读全文
相关推荐
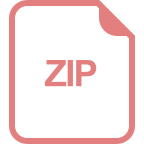
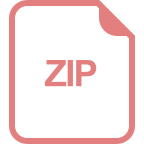
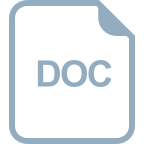
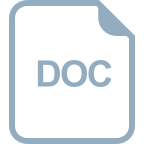
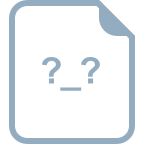
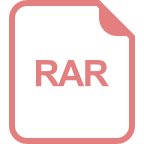
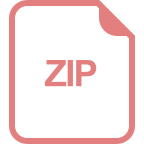
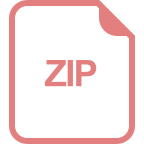
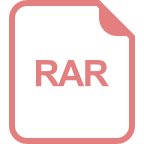
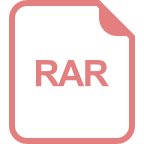
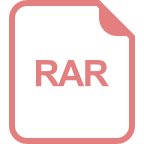
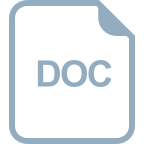
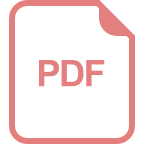
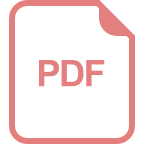
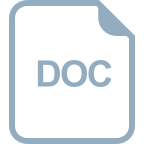
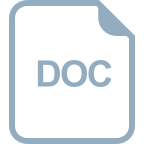