重载当前vue组件
时间: 2023-07-23 16:20:08 浏览: 164
要重载当前 Vue 组件,可以使用 `this.$forceUpdate()` 方法强制更新当前组件。具体步骤如下:
1. 在组件中调用 `$forceUpdate()` 方法:
```
export default {
methods: {
reloadComponent() {
this.$forceUpdate()
}
}
}
```
2. 在页面中调用组件的方法:
```
<template>
<div>
<my-component ref="myComponent"></my-component>
<button @click="reloadComponent">重载组件</button>
</div>
</template>
<script>
import MyComponent from './MyComponent.vue'
export default {
components: {
MyComponent
},
methods: {
reloadComponent() {
this.$refs.myComponent.reloadComponent()
}
}
}
</script>
```
这样就可以在页面上通过点击按钮重载当前 Vue 组件了。需要注意的是,如果修改了组件的选项,必须调用 `$forceUpdate()` 方法才能使修改生效。
相关问题
vite vue3点击浏览器刷新 重载当前组件
Vite是一个快速的前端构建工具,特别适合Vue 3项目。当你使用Vite配合Vue 3,并且遇到点击浏览器刷新时组件重新加载的问题,这通常是因为Vite采用了即时热更新(Hot Module Replacement, HMR)特性。HMR允许你在开发过程中修改代码后几乎立即看到变化,无需完整地刷新页面。
为了实现在点击刷新时仅重载当前组件而不是整个应用,你需要确保以下几个步骤:
1. **配置Vite**:确保你的Vite.config.js文件正确设置了`build.rollupOptions.watch`和`hotModuleReplacement`选项,这样Vite才能启用热更新功能。
```javascript
import { defineConfig } from 'vite';
import vue from '@vitejs/plugin-vue';
export default defineConfig({
plugins: [vue()],
build: {
rollupOptions: {
input: ['src/main.js'],
watch: {
clearScreen: false, // 避免每次刷新都清屏
},
hotModuleReplacement: true, // 启用热模块替换
},
},
});
```
2. **路由配置**:如果你使用的是Vue Router,确保你的组件路径和路由配置对应。当用户点击刷新时,如果没有其他路由改变,Vite应该能识别并只更新相关的组件。
3. **组件内部处理**:确保你的组件内有适当的生命周期钩子(如`beforeDestroy`),以便在组件卸载前清理资源,减少不必要的刷新影响。
4. **避免全局状态污染**:全局状态可能导致热更新失败。尽量使用局部状态或者Vuex等状态管理库来存储数据。
5. **检查开发环境设置**:确认你在开发模式下(非生产模式)运行应用,因为生产环境中默认禁用了HMR。
vue 重载当前路由
Vue.js 中,重载当前路由通常是指当你需要改变某个组件的内容而不刷新整个页面时,可以在组件内或者全局路由守卫中实现动态加载新的内容。这通过 `this.$router.push()` 或者 `this.$router.replace()` 方法结合动态参数来完成。
例如,在一个单文件组件 (Single File Component, SFC) 中,你可以这样做:
```javascript
methods: {
loadNewContent() {
const newRoute = { name: 'yourRouteName', params: { id: yourNewId } };
this.$router.push(newRoute);
}
}
```
然后在模板里添加一个按钮或者事件监听器,触发 `loadNewContent` 方法:
```html
<button @click="loadNewContent">切换内容</button>
```
如果你想替换当前路由而不是添加一个新的历史记录,可以使用 `replace`:
```javascript
this.$router.replace({ ...newRoute });
```
阅读全文
相关推荐
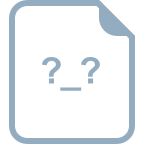

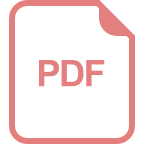
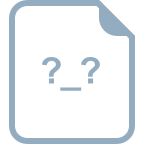
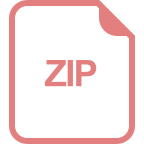
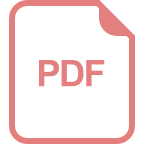
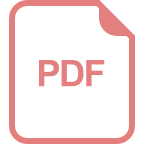
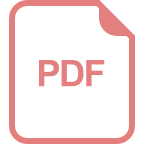
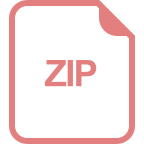
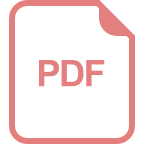
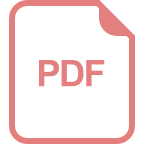
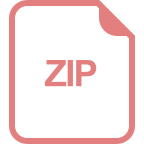
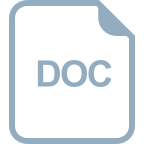
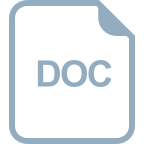
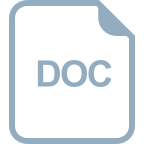
