在安卓开发中 图片工具类 应该如何封装? 代码
时间: 2024-03-22 09:37:22 浏览: 102
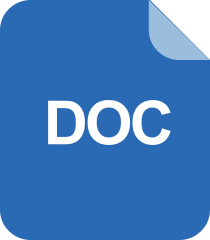
android中对图片处理封装的一些方法

以下是一个简单的安卓图片工具类的代码示例:
```java
public class ImageUtils {
/**
* 压缩图片
*
* @param imagePath 图片路径
* @param targetWidth 目标宽度
* @param targetHeight 目标高度
* @param quality 压缩质量
* @return 压缩后的图片
*/
public static Bitmap compressImage(String imagePath, int targetWidth, int targetHeight, int quality) {
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeFile(imagePath, options);
int width = options.outWidth;
int height = options.outHeight;
int sampleSize = 1;
if (width > targetWidth || height > targetHeight) {
int widthRatio = Math.round((float) width / (float) targetWidth);
int heightRatio = Math.round((float) height / (float) targetHeight);
sampleSize = Math.min(widthRatio, heightRatio);
}
options.inJustDecodeBounds = false;
options.inSampleSize = sampleSize;
Bitmap bitmap = BitmapFactory.decodeFile(imagePath, options);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.JPEG, quality, outputStream);
byte[] bytes = outputStream.toByteArray();
return BitmapFactory.decodeByteArray(bytes, 0, bytes.length);
}
/**
* 缩放图片
*
* @param bitmap 原始图片
* @param targetWidth 目标宽度
* @param targetHeight 目标高度
* @return 缩放后的图片
*/
public static Bitmap scaleImage(Bitmap bitmap, int targetWidth, int targetHeight) {
int width = bitmap.getWidth();
int height = bitmap.getHeight();
float scaleWidth = ((float) targetWidth) / width;
float scaleHeight = ((float) targetHeight) / height;
Matrix matrix = new Matrix();
matrix.postScale(scaleWidth, scaleHeight);
return Bitmap.createBitmap(bitmap, 0, 0, width, height, matrix, true);
}
/**
* 裁剪图片
*
* @param bitmap 原始图片
* @param startX 裁剪起始 X 坐标
* @param startY 裁剪起始 Y 坐标
* @param width 裁剪宽度
* @param height 裁剪高度
* @return 裁剪后的图片
*/
public static Bitmap cropImage(Bitmap bitmap, int startX, int startY, int width, int height) {
return Bitmap.createBitmap(bitmap, startX, startY, width, height);
}
/**
* 旋转图片
*
* @param bitmap 原始图片
* @param degrees 旋转角度
* @return 旋转后的图片
*/
public static Bitmap rotateImage(Bitmap bitmap, float degrees) {
Matrix matrix = new Matrix();
matrix.postRotate(degrees);
return Bitmap.createBitmap(bitmap, 0, 0, bitmap.getWidth(), bitmap.getHeight(), matrix, true);
}
/**
* 添加水印
*
* @param bitmap 原始图片
* @param watermark 水印图片
* @return 添加水印后的图片
*/
public static Bitmap addWatermark(Bitmap bitmap, Bitmap watermark) {
int width = bitmap.getWidth();
int height = bitmap.getHeight();
Bitmap result = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(result);
canvas.drawBitmap(bitmap, 0, 0, null);
canvas.drawBitmap(watermark, width - watermark.getWidth(), height - watermark.getHeight(), null);
return result;
}
/**
* 根据路径获取图片
*
* @param imagePath 图片路径
* @return 图片
*/
public static Bitmap getBitmapFromPath(String imagePath) {
return BitmapFactory.decodeFile(imagePath);
}
/**
* 将图片转换为字节数组
*
* @param bitmap 图片
* @return 字节数组
*/
public static byte[] bitmapToBytes(Bitmap bitmap) {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.PNG, 100, outputStream);
return outputStream.toByteArray();
}
/**
* 将字节数组转换为图片
*
* @param bytes 字节数组
* @return 图片
*/
public static Bitmap bytesToBitmap(byte[] bytes) {
return BitmapFactory.decodeByteArray(bytes, 0, bytes.length);
}
/**
* 将图片转换为 Base64 编码的字符串
*
* @param bitmap 图片
* @return Base64 编码的字符串
*/
public static String bitmapToBase64(Bitmap bitmap) {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.PNG, 100, outputStream);
byte[] bytes = outputStream.toByteArray();
return Base64.encodeToString(bytes, Base64.DEFAULT);
}
/**
* 将 Base64 编码的字符串转换为图片
*
* @param base64 Base64 编码的字符串
* @return 图片
*/
public static Bitmap base64ToBitmap(String base64) {
byte[] bytes = Base64.decode(base64, Base64.DEFAULT);
return BitmapFactory.decodeByteArray(bytes, 0, bytes.length);
}
}
```
这里封装了常用的图片处理功能,包括压缩、缩放、裁剪、旋转、添加水印、转换为字节数组和转换为 Base64 编码的字符串等。每个方法都有相应的参数和返回值,以便于使用。
当然,这只是一个简单的示例,实际上图片处理功能可能更加复杂和多样化,需要根据具体需求进行封装。同时,还需要考虑到性能和效率等因素,以保证应用的流畅性和响应速度。
阅读全文
相关推荐

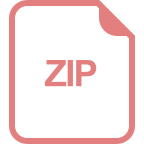
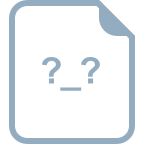
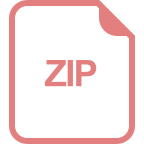
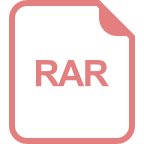
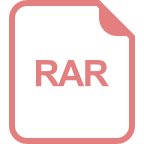
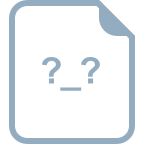
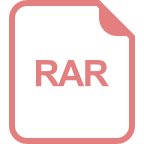
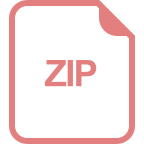
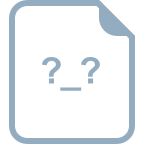
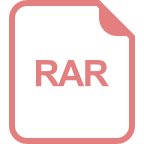
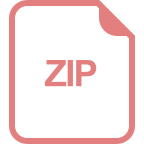
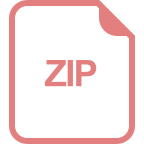
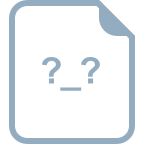
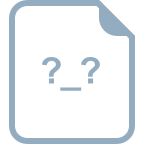