sprimgboot实现点赞功能代码实现
时间: 2023-09-01 14:13:42 浏览: 101
下面是一个简单的 Spring Boot 实现点赞功能的示例代码:
1. 点赞实体类
```java
@Entity
public class Like {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Long userId;
private Long targetId;
private LocalDateTime createTime;
// getter 和 setter 略
}
```
2. 点赞服务
```java
@Service
public class LikeService {
@Autowired
private LikeRepository likeRepository;
public void like(Long userId, Long targetId) {
Like like = new Like();
like.setUserId(userId);
like.setTargetId(targetId);
like.setCreateTime(LocalDateTime.now());
likeRepository.save(like);
}
public void unlike(Long userId, Long targetId) {
likeRepository.deleteByUserIdAndTargetId(userId, targetId);
}
public Long count(Long targetId) {
return likeRepository.countByTargetId(targetId);
}
}
```
3. 点赞控制器
```java
@RestController
@RequestMapping("/like")
public class LikeController {
@Autowired
private LikeService likeService;
@PostMapping("/like")
public void like(@RequestParam Long userId, @RequestParam Long targetId) {
likeService.like(userId, targetId);
}
@PostMapping("/unlike")
public void unlike(@RequestParam Long userId, @RequestParam Long targetId) {
likeService.unlike(userId, targetId);
}
@GetMapping("/count")
public Long count(@RequestParam Long targetId) {
return likeService.count(targetId);
}
}
```
4. 前端页面
```html
<div>
<button id="likeButton" onclick="like()">点赞</button>
<span id="likeCount"></span>
</div>
<script>
function like() {
$.ajax({
type: "POST",
url: "/like/like",
data: {
userId: "1",
targetId: "1001"
},
success: function() {
$("#likeButton").attr("disabled", true);
countLike();
}
});
}
function unlike() {
$.ajax({
type: "POST",
url: "/like/unlike",
data: {
userId: "1",
targetId: "1001"
},
success: function() {
$("#likeButton").attr("disabled", false);
countLike();
}
});
}
function countLike() {
$.ajax({
type: "GET",
url: "/like/count",
data: {
targetId: "1001"
},
success: function(count) {
$("#likeCount").text(count);
}
});
}
$(document).ready(function() {
countLike();
});
</script>
```
以上代码实现了一个简单的点赞功能,用户点击点赞按钮后,调用 AJAX 请求将点赞信息发送到后端控制器,然后后端服务将点赞信息保存到数据库中,并且更新点赞数的显示。如果用户取消点赞,则发送取消点赞请求,后端服务将相应的点赞信息从数据库中删除,并且更新点赞数的显示。
阅读全文
相关推荐

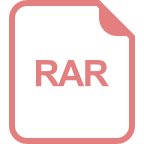




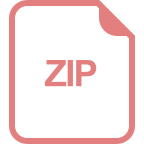
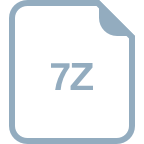
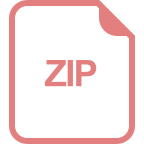
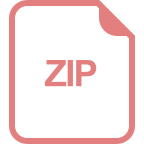
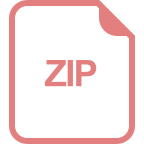