GORM SELECT * FROM table1 WHERE type = '图片'UNIONSELECT * FROM table2 WHERE type = '图片'UNIONSELECT * FROM table3 WHERE type = '图片'UNIONSELECT * FROM table4 WHERE type = '图片'UNIONSELECT * FROM table5 WHERE type = '图片';
时间: 2024-01-04 17:02:34 浏览: 141
以上 SQL 语句是一个查询语句,目的是从 5 个表中选出 type 字段为 '图片' 的所有行,并将它们合并成一个结果集。其中使用了 UNION 运算符将多个 SELECT 语句的结果合并。这里的 * 表示选取所有列。GORM 是一个 Go 语言 ORM 框架,可以通过该框架进行数据库操作,包括查询、插入、更新、删除等。在 GORM 中,可以使用 Raw 方法执行原始 SQL 语句,例如:
```
db.Raw("SELECT * FROM table1 WHERE type = ? UNION SELECT * FROM table2 WHERE type = ? UNION SELECT * FROM table3 WHERE type = ? UNION SELECT * FROM table4 WHERE type = ? UNION SELECT * FROM table5 WHERE type = ?", "图片", "图片", "图片", "图片", "图片").Scan(&result)
```
其中,? 是占位符,用于占据 SQL 语句中的参数位置,Scan 方法用于将查询结果转换为指定类型的数据结构。
相关问题
改进代码var req []*Total res := db.Scopes(models.Paginate(page, pageSize)).Raw("SELECT * FROM flaws WHERE facility_id = ? "+ "UNION SELECT * FROM issues WHERE facility_id = ? "+ "UNION SELECT * FROM records WHERE facility_id = ? "+ "UNION SELECT * FROM qualities WHERE facility_id = ? "+ "UNION SELECT * FROM inspections WHERE facility_id = ?", code, code, code, code, code).Find(&req)
这段代码可以优化为使用GORM的联合查询(Union)功能,避免使用Raw SQL语句。
可以尝试这样改进代码:
```
err := db.Where("facility_id = ?", code).
Select("id, created_at, updated_at, field1, field2, NULL as field3, NULL as field4, NULL as field5").
Table("flaws").
Union(db.Where("facility_id = ?", code).
Select("id, created_at, updated_at, field1, NULL as field2, field3, field4, NULL as field5").
Table("issues")).
Union(db.Where("facility_id = ?", code).
Select("id, created_at, updated_at, field1, NULL as field2, NULL as field3, NULL as field4, field5").
Table("records")).
Union(db.Where("facility_id = ?", code).
Select("id, created_at, updated_at, field1, field2, NULL as field3, NULL as field4, NULL as field5").
Table("qualities")).
Union(db.Where("facility_id = ?", code).
Select("id, created_at, updated_at, field1, NULL as field2, field3, field4, field5").
Table("inspections")).
Order("created_at desc").
Limit(pageSize).
Offset((page - 1) * pageSize).
Find(&req).Error
```
在这个示例中,我们使用了 `Select` 函数选择每个表中的字段,并将不需要的字段设置为 `NULL`。然后我们使用 `Union` 函数将所有查询合并到一起,并使用 `Order`、`Limit` 和 `Offset` 函数进行分页和排序。
这种方法比使用 `Raw` 函数更安全,因为它可以防止 SQL 注入攻击,并且更易于维护。
改进代码var req []*Total res := db.Scopes(models.Paginate(page, pageSize)).Raw("SELECT * FROM flaws WHERE facility_id = ? "+ "UNION SELECT * FROM issues WHERE facility_id = ? "+ "UNION SELECT * FROM records WHERE facility_id = ? "+ "UNION SELECT * FROM qualities WHERE facility_id = ? "+ "UNION SELECT * FROM inspections WHERE facility_id = ?", code, code, code, code, code).Find(&req) if res.RowsAffected == 0 { resp.Code = 400 resp.Msg = "记录不存在" ctx.JSON(400, resp) return } resp.Data = req ctx.JSON(200, resp)
在这段代码的改进中,你可以使用GORM的“Preload”函数,将查询分成多个独立的查询,以便更好地管理和优化查询,并且可以避免使用Raw SQL语句。下面是一个示例代码:
```
var flaws []*models.Flaw
var issues []*models.Issue
var records []*models.Record
var qualities []*models.Quality
var inspections []*models.Inspection
if err := db.Where("facility_id = ?", code).Preload("Facility").Limit(pageSize).Offset((page - 1) * pageSize).Find(&flaws).Error; err != nil {
resp.Code = 400
resp.Msg = "查询记录失败"
ctx.JSON(400, resp)
return
}
if len(flaws) == 0 {
resp.Code = 400
resp.Msg = "记录不存在"
ctx.JSON(400, resp)
return
}
if err := db.Where("facility_id = ?", code).Preload("Facility").Limit(pageSize).Offset((page - 1) * pageSize).Find(&issues).Error; err != nil {
resp.Code = 400
resp.Msg = "查询记录失败"
ctx.JSON(400, resp)
return
}
if err := db.Where("facility_id = ?", code).Preload("Facility").Limit(pageSize).Offset((page - 1) * pageSize).Find(&records).Error; err != nil {
resp.Code = 400
resp.Msg = "查询记录失败"
ctx.JSON(400, resp)
return
}
if err := db.Where("facility_id = ?", code).Preload("Facility").Limit(pageSize).Offset((page - 1) * pageSize).Find(&qualities).Error; err != nil {
resp.Code = 400
resp.Msg = "查询记录失败"
ctx.JSON(400, resp)
return
}
if err := db.Where("facility_id = ?", code).Preload("Facility").Limit(pageSize).Offset((page - 1) * pageSize).Find(&inspections).Error; err != nil {
resp.Code = 400
resp.Msg = "查询记录失败"
ctx.JSON(400, resp)
return
}
var req []*models.Total
for _, f := range flaws {
req = append(req, &models.Total{Facility: f.Facility, Flaw: f})
}
for _, i := range issues {
req = append(req, &models.Total{Facility: i.Facility, Issue: i})
}
for _, r := range records {
req = append(req, &models.Total{Facility: r.Facility, Record: r})
}
for _, q := range qualities {
req = append(req, &models.Total{Facility: q.Facility, Quality: q})
}
for _, insp := range inspections {
req = append(req, &models.Total{Facility: insp.Facility, Inspection: insp})
}
resp.Data = req
ctx.JSON(200, resp)
```
在这个改进后的代码中,我们使用了GORM的“Preload”函数,将每个表的数据分别加载到单独的数组中,最后将这些数组合并到一个“Total”数组中。这样就可以有效地管理和优化查询,并且不需要使用Raw SQL语句。
阅读全文
相关推荐
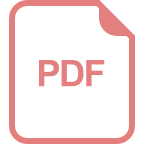
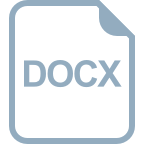














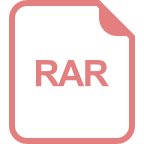