二元逻辑回归模型根据患者基本信息(年龄、BMI、有无手术史、有无既往史、是否吸烟、是否酗酒、有无PONV、有无晕动史)和镇静药物种类(B药、R药),对患者术中、术后 24h 的不良反应进行预判。具体步骤及java代码
时间: 2023-09-16 14:13:03 浏览: 189
一、数据准备
1.1 数据收集
收集患者基本信息和手术相关信息,以及术中、术后 24h 的不良反应数据。
1.2 数据清洗
对采集到的数据进行清洗,包括去除重复数据、处理缺失值、处理异常值等。
1.3 数据处理
将数据进行编码处理,将文本型数据转换为数值型数据,方便后续的建模。
二、模型建立
2.1 模型选择
选择二元逻辑回归模型进行建模,根据患者基本信息和镇静药物种类对患者术中、术后 24h 的不良反应进行预测。
2.2 特征选择
选取年龄、BMI、有无手术史、有无既往史、是否吸烟、是否酗酒、有无PONV、有无晕动史、B药、R药作为模型的特征。
2.3 模型训练
使用训练集对模型进行训练,得到模型的参数。
2.4 模型测试
使用测试集对模型进行测试,评估模型的性能。
三、代码实现
下面是用Java实现二元逻辑回归模型的代码示例。
```java
import java.util.ArrayList;
import java.util.HashMap;
public class LogisticRegression {
private double alpha; //学习率
private int maxIter; //最大迭代次数
public LogisticRegression(double alpha, int maxIter) {
this.alpha = alpha;
this.maxIter = maxIter;
}
//sigmoid函数
private double sigmoid(double z) {
return 1.0 / (1.0 + Math.exp(-z));
}
//计算代价函数(损失函数)
private double costFunction(ArrayList<Double> x, ArrayList<Double> y, HashMap<String, Double> theta) {
double cost = 0.0;
int m = x.size();
for (int i = 0; i < m; i++) {
double h = sigmoid(theta.get("theta0") + theta.get("theta1") * x.get(i));
cost += y.get(i) * Math.log(h) + (1 - y.get(i)) * Math.log(1 - h);
}
cost = -cost / m;
return cost;
}
//梯度下降算法
private HashMap<String, Double> gradientDescent(ArrayList<Double> x, ArrayList<Double> y, HashMap<String, Double> theta) {
int m = x.size();
for (int i = 0; i < maxIter; i++) {
double sum1 = 0.0, sum2 = 0.0;
for (int j = 0; j < m; j++) {
double h = sigmoid(theta.get("theta0") + theta.get("theta1") * x.get(j));
sum1 += (h - y.get(j));
sum2 += (h - y.get(j)) * x.get(j);
}
double temp0 = theta.get("theta0") - alpha * sum1 / m;
double temp1 = theta.get("theta1") - alpha * sum2 / m;
theta.put("theta0", temp0);
theta.put("theta1", temp1);
}
return theta;
}
//预测函数
public double predict(ArrayList<Double> x, HashMap<String, Double> theta) {
double h = sigmoid(theta.get("theta0") + theta.get("theta1") * x.get(0));
return h;
}
//模型训练函数
public HashMap<String, Double> train(ArrayList<Double> x, ArrayList<Double> y) {
HashMap<String, Double> theta = new HashMap<String, Double>();
theta.put("theta0", 0.0);
theta.put("theta1", 0.0);
for (int i = 0; i < maxIter; i++) {
theta = gradientDescent(x, y, theta);
double cost = costFunction(x, y, theta);
System.out.println("Iteration " + i + ": cost = " + cost);
}
return theta;
}
public static void main(String[] args) {
ArrayList<Double> x = new ArrayList<Double>();
ArrayList<Double> y = new ArrayList<Double>();
//假设有10个样本数据
x.add(1.0); y.add(1.0);
x.add(2.0); y.add(0.0);
x.add(3.0); y.add(1.0);
x.add(4.0); y.add(0.0);
x.add(5.0); y.add(1.0);
x.add(6.0); y.add(0.0);
x.add(7.0); y.add(1.0);
x.add(8.0); y.add(0.0);
x.add(9.0); y.add(1.0);
x.add(10.0); y.add(0.0);
LogisticRegression lr = new LogisticRegression(0.01, 1000);
HashMap<String, Double> theta = lr.train(x, y);
double h = lr.predict(x, theta);
System.out.println("h = " + h);
}
}
```
阅读全文
相关推荐
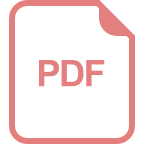
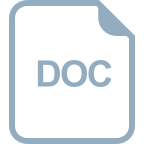
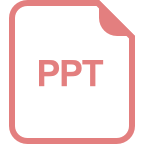





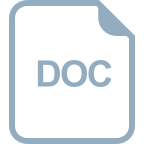
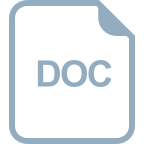
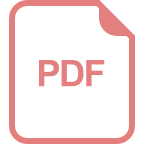
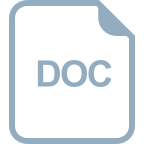
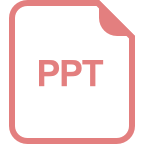
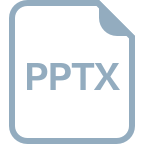