Android compose
时间: 2023-11-02 21:48:59 浏览: 50
Android Compose是一个用于构建Android用户界面的现代化工具包。它使用Kotlin编写,具有声明式UI编写方式,可以帮助开发者更轻松地构建复杂的UI界面。相比传统的Android布局方式,Android Compose提供了更加灵活和快速的UI编写方式,同时还提供了一些强大的工具和组件,如可组合式UI、状态管理、动画效果等。Android Compose目前还处于预览版,但是它已经受到了广泛的关注和使用。
相关问题
android compose
Android Compose是一种用于构建用户界面的声明式UI框架。它允许开发者使用Kotlin编写简洁、可组合和可测试的UI代码。下面是使用Android Compose的步骤:
1. 在项目的build.gradle文件中添加Compose依赖:
```groovy
dependencies {
implementation 'androidx.compose.ui:ui:1.0.0-beta01'
implementation 'androidx.compose.material:material:1.0.0-beta01'
implementation 'androidx.compose.runtime:runtime:1.0.0-beta01'
}
```
2. 在Activity中使用ComposeView来关联Compose代码和传统的Android View:
```kotlin
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.compose.foundation.Text
import androidx.compose.foundation.layout.Column
import androidx.compose.runtime.Composable
import androidx.compose.ui.platform.setContent
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ComposeContent()
}
}
}
@Composable
fun ComposeContent() {
Column {
Text(text = "Hello, Android Compose!")
}
}
```
3. 运行项目,即可看到使用Android Compose构建的界面。
android compose 蓝牙
Android Compose是一种用于构建用户界面的现代化工具包,而蓝牙是一种用于在不同设备之间进行无线通信的技术。在Android Compose中使用蓝牙可以实现一些有趣和有用的功能。
首先,Android Compose可以让我们更容易地创建具有蓝牙功能的应用程序界面。通过使用Compose的声明式语法,我们可以轻松地在应用程序中添加蓝牙设备的连接和控制功能。这使得开发者更容易实现与蓝牙相关的功能,如扫描蓝牙设备、连接设备、发送和接收数据等。
其次,使用Android Compose能够提供更好的用户体验。通过使用Composable函数和状态管理,我们可以实时更新UI以显示蓝牙设备的连接状态,包括设备是否已连接、连接时的信号强度等。这使得用户能够更好地了解蓝牙设备的状态,例如是否已成功连接,从而增强了用户体验。
另外,Android Compose还可以简化蓝牙设备的管理和控制。我们可以使用Compose来创建蓝牙设备的列表,并通过点击列表项来连接设备。同时,Compose还提供了一种简洁的方式来发送和接收数据,使得开发者更容易实现蓝牙通信功能。
总之,Android Compose为开发者提供了更便捷和灵活的方式来构建具有蓝牙功能的应用程序界面。通过使用Compose,开发者可以更容易地实现与蓝牙设备的通信,并提供更好的用户体验。这使得蓝牙技术在Android应用程序开发中变得更加普遍和易用。
相关推荐
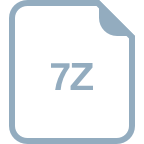
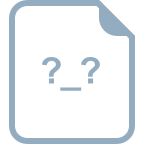












