用flask写一个四则运算web
时间: 2024-06-08 19:05:30 浏览: 90
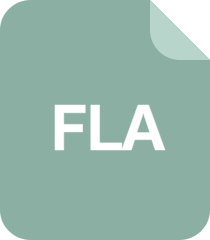
flash 四则运算

好的,以下是一个简单的四则运算的 Flask web 应用:
```python
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/calculate', methods=['POST'])
def calculate():
error = None
try:
num1 = int(request.form['num1'])
num2 = int(request.form['num2'])
operator = request.form['operator']
if operator == '+':
result = num1 + num2
elif operator == '-':
result = num1 - num2
elif operator == '*':
result = num1 * num2
elif operator == '/':
result = num1 / num2
else:
error = 'Invalid operator'
return render_template('index.html', error=error)
except:
error = 'Invalid input'
return render_template('index.html', error=error)
return render_template('result.html', result=result)
if __name__ == '__main__':
app.run(debug=True)
```
这个应用有两个页面,一个是主页 `index.html`,另一个是结果页面 `result.html`。主页中有一个表单用于输入两个数字和一个运算符,提交表单后会跳转到结果页面显示计算结果。
以下是主页 `index.html` 的 HTML 代码:
```html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>四则运算</title>
</head>
<body>
{% if error %}
<p style="color: red;">{{ error }}</p>
{% endif %}
<form method="post" action="/calculate">
<input type="number" name="num1" required>
<select name="operator" required>
<option value="+">+</option>
<option value="-">-</option>
<option value="*">*</option>
<option value="/">/</option>
</select>
<input type="number" name="num2" required>
<input type="submit" value="计算">
</form>
</body>
</html>
```
在这个页面中,我们使用了 Flask 的模板引擎来渲染页面。如果有错误信息,会在页面中显示红色文字。表单中有三个输入框,分别用于输入两个数字和一个运算符。
以下是结果页面 `result.html` 的 HTML 代码:
```html
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>计算结果</title>
</head>
<body>
<p>计算结果:{{ result }}</p>
</body>
</html>
```
在结果页面中,我们使用了 Flask 的模板引擎来渲染页面。页面中会显示计算结果。
使用 Flask 运行这个应用,打开浏览器访问 `http://localhost:5000/` 可以看到主页。
注意:这个应用只是一个简单的演示,没有对用户输入进行严格的校验和异常处理,实际应用中需要更加严格地处理用户输入和错误情况。
阅读全文
相关推荐
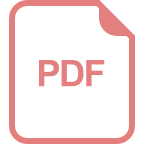
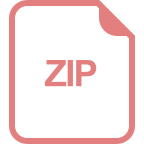
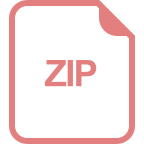
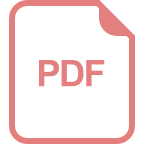
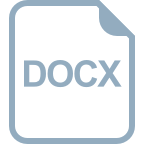
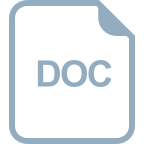
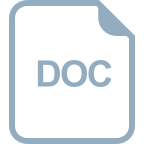
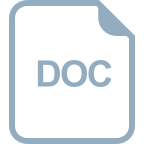
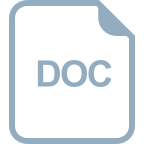
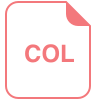
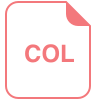
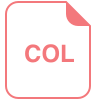
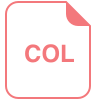
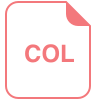
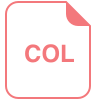
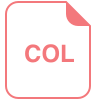
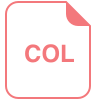