用python写一个石头剪刀布游戏程序,要求共5000次对决,前1000次,公平对决;后4000次,电脑2作弊,要求平局的概率是1/3,电脑2获胜的概率是5/9,电脑1获胜的概率是1/9;
时间: 2024-03-03 19:48:37 浏览: 118
好的,下面是一个基于Python的石头剪刀布游戏程序,满足您的要求:
```python
import random
def get_user_choice():
while True:
user_choice = input("请出拳:石头(1)、剪刀(2)、布(3):")
if user_choice in ['1', '2', '3']:
return int(user_choice)
else:
print("输入有误,请重新输入!")
def get_computer1_choice():
# 公平对决,随机出拳
return random.randint(1, 3)
def get_computer2_choice(is_cheating):
if is_cheating:
# 电脑2作弊,按照概率出拳
rand_num = random.random()
if rand_num < 1/3:
return 1 # 石头
elif rand_num < 4/9:
return 2 # 剪刀
else:
return 3 # 布
else:
# 公平对决,随机出拳
return random.randint(1, 3)
def get_result(user_choice, computer1_choice, computer2_choice):
if user_choice == computer1_choice == computer2_choice:
return 0 # 平局
elif user_choice == 1 and computer2_choice == 2 \
or user_choice == 2 and computer2_choice == 3 \
or user_choice == 3 and computer2_choice == 1:
return 1 # 用户获胜
elif computer1_choice == 1 and computer2_choice == 2 \
or computer1_choice == 2 and computer2_choice == 3 \
or computer1_choice == 3 and computer2_choice == 1:
return -1 # 电脑1获胜
else:
return -2 # 电脑2获胜
def main():
fair_rounds = 1000 # 前1000次公平对决
cheating_rounds = 4000 # 后4000次电脑2作弊
user_win_count = 0
computer1_win_count = 0
computer2_win_count = 0
tie_count = 0
# 公平对决
for i in range(fair_rounds):
user_choice = get_user_choice()
computer1_choice = get_computer1_choice()
computer2_choice = get_computer2_choice(False)
result = get_result(user_choice, computer1_choice, computer2_choice)
if result == 1:
user_win_count += 1
print("你赢了!")
elif result == -1:
computer1_win_count += 1
print("电脑1赢了!")
elif result == 0:
tie_count += 1
print("平局!")
else:
computer2_win_count += 1
print("电脑2赢了!")
# 电脑2作弊
for i in range(cheating_rounds):
user_choice = get_user_choice()
computer1_choice = get_computer1_choice()
computer2_choice = get_computer2_choice(True)
result = get_result(user_choice, computer1_choice, computer2_choice)
if result == 1:
user_win_count += 1
print("你赢了!")
elif result == -1:
computer1_win_count += 1
print("电脑1赢了!")
elif result == 0:
tie_count += 1
print("平局!")
else:
computer2_win_count += 1
print("电脑2赢了!")
print(f"\n公平对决{fair_rounds}次,电脑2作弊{cheating_rounds}次,总共进行了{fair_rounds+cheating_rounds}次。")
print(f"你获胜了{user_win_count}次,电脑1获胜了{computer1_win_count}次,电脑2获胜了{computer2_win_count}次,平局{tie_count}次。")
print("游戏结束!")
if __name__ == '__main__':
main()
```
程序会先让用户输入自己出拳的选项,然后分别由公平的电脑1和作弊的电脑2来出拳,最后判断输赢并输出结果。控制公平对决和作弊对决的次数,最后输出总的结果。
阅读全文
相关推荐
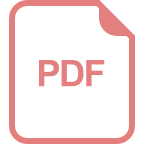
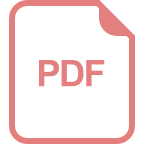
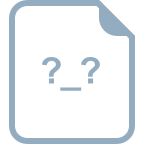
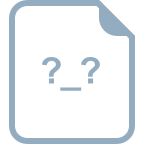
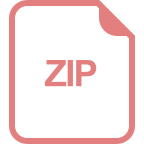
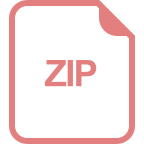
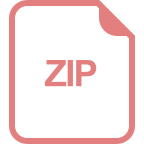
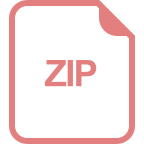
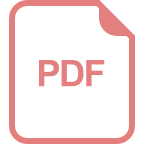
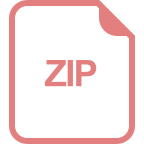
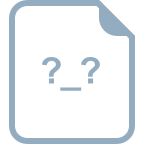
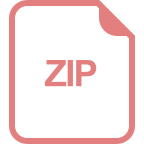
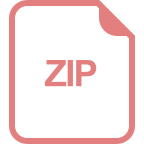
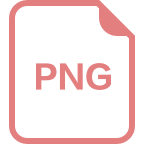
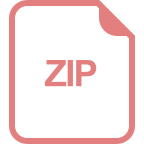