字符串转换为宽字符类型
时间: 2024-05-05 09:20:47 浏览: 175
要将字符串转换为宽字符类型,可以使用 C++ 标准库中的 wstring 类型。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <locale>
int main() {
std::string str = "Hello, world!"; // 定义一个字符串
std::wstring_convert<std::codecvt_utf8_utf16<wchar_t>> converter; // 定义一个转换器
std::wstring wstr = converter.from_bytes(str); // 将字符串转换为宽字符串
std::wcout << wstr << std::endl; // 输出宽字符串
return 0;
}
```
在这个示例中,我们使用了 `std::wstring_convert` 类型并传递了一个模板参数 `std::codecvt_utf8_utf16<wchar_t>`,该参数指定了要使用的字符编码转换器。我们还使用 `from_bytes` 函数将字符串转换为宽字符串,并使用 `std::wcout` 输出它。
相关问题
将const char*类型的字符串转换为LPCTSTR类型
将const char*类型的字符串转换为LPCTSTR类型的字符串,可以使用宏定义TCHAR和相关的字符串转换函数。
例如,以下代码将const char*类型的字符串转换为LPCTSTR类型的字符串:
```
const char* cstr = "hello";
int len = strlen(cstr) + 1;
int size = MultiByteToWideChar(CP_ACP, 0, cstr, len, NULL, 0);
wchar_t* wstr = new wchar_t[size];
MultiByteToWideChar(CP_ACP, 0, cstr, len, wstr, size);
LPCTSTR lpStr = wstr;
delete[] wstr;
```
这里使用了MultiByteToWideChar函数来将const char*类型的字符串转换为宽字符类型的字符串(wchar_t*),然后再将宽字符类型的字符串转换为LPCTSTR类型的字符串。注意要在使用完宽字符类型的字符串后将其释放。
如何将宽字符字符串转换成一个无符号整型数unsigned int
可以使用 C++ 标准库中的 std::stoul 函数将宽字符字符串转换成一个无符号整型数 unsigned int。该函数的声明如下:
```c++
unsigned long stoul( const wstring& str, size_t* pos = 0, int base = 10 );
```
其中,str 是要转换的宽字符字符串;pos 是一个指向 size_t 类型变量的指针,用于存储转换结束的位置(如果不需要知道转换结束的位置,可以将该参数设置为 nullptr);base 是进制数,可以是 2 到 36 之间的任意值。函数返回转换后的无符号整型数 unsigned int。
示例代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main()
{
wstring str = L"12345";
unsigned int num = stoul(str);
cout << "num = " << num << endl;
return 0;
}
```
输出结果为:
```
num = 12345
```
阅读全文
相关推荐
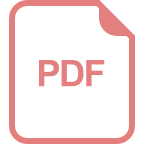
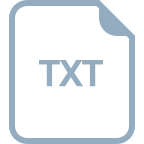
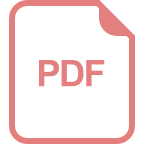
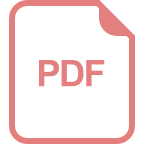
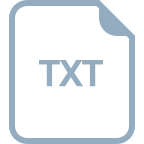
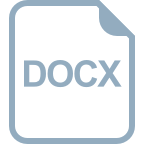
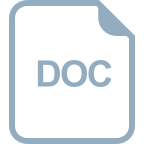
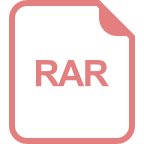
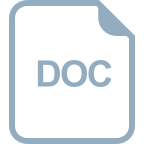
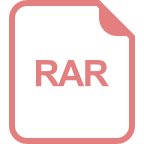
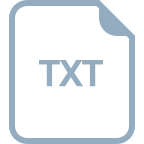
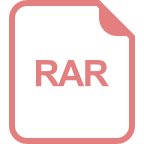
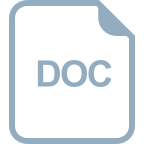
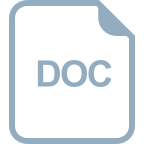
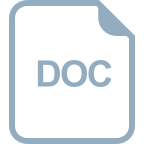