编写一个计算个人所得税程序。设某人月收入为x元,假设个人所得税征收方法如下: 当500<x≤1000时,应征税为(x-500)*5%;当1000<x≤2000时,应征税为(x-500)*10%; 当2000<x≤5000时,应征税为(x-500)*15%;当5000<x≤20000时,应征税为(x-500)*20%; 当20000<x时,应征税为(x-500)*30% 分别利用if语句和switch语句来实现。
时间: 2023-05-24 08:04:20 浏览: 98
Null is a term used to describe a lack of value or absence of any data. It represents the absence of any meaningful or significant worth or value. It is often seen as an empty or blank value, as it does not represent any specific number, quantity, or object. In computer programming, null is often used to indicate that a variable or object does not have a valid value or is not initialized. It is a commonly used value in programming languages like Java, C++, Python, and many others.
相关问题
python个人所得税编写一个计算个人所得税的程序,要求输入收入金额后,能够输出应缴的个人所得税。个人所得税征收办法如下起征点为2000元。不超过500元的部分,征收5%。超过500~2000元的部分,征收10%。超超过2000~5000元的部分,征收15%。超过5000~20000元的部分,征收20%。超过20000~40000元的部分,征收25%。超过40000~60000元的部分,征收30%。超过60000~80000元的部分,征收35%。超过征收40%。超过80000~100000元以上的,征收45%。
好的,可以使用以下代码实现:
```python
income = float(input("请输入收入金额:"))
if income <= 2000:
tax = 0
elif income <= 5000:
tax = (income - 2000) * 0.05
elif income <= 20000:
tax = 300 + (income - 5000) * 0.1
elif income <= 40000:
tax = 2300 + (income - 20000) * 0.15
elif income <= 60000:
tax = 5300 + (income - 40000) * 0.2
elif income <= 80000:
tax = 9300 + (income - 60000) * 0.25
elif income <= 100000:
tax = 15300 + (income - 80000) * 0.3
else:
tax = 21300 + (income - 100000) * 0.35
print("应缴个人所得税为:", tax, "元")
```
使用 `input()` 函数从键盘上获取用户输入的收入金额,并将其保存在变量 `income` 中。然后,使用 `if...elif...else` 语句根据不同的收入金额计算应缴个人所得税,并将结果保存在变量 `tax` 中。最后,使用 `print()` 函数输出结果。
需要注意的是,需要使用浮点数来处理收入金额和个人所得税,否则会出现计算错误。
JAVA计算个人所得税
JAVA计算个人所得税是一种使用JAVA语言编写的程序,可以根据个人月收入计算出应缴纳的个人所得税。根据个人所得税征收方法,该程序可以分别计算出不同收入范围内应缴纳的税款金额。通过输入个人月收入,程序可以自动计算出应缴纳的个人所得税金额。该程序可以帮助人们更方便地计算个人所得税,提高计算的准确性和效率。
相关推荐
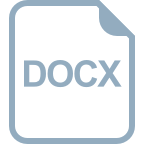












