linux C线程消息机制
时间: 2023-10-26 15:32:32 浏览: 112
Linux C线程间通信的方式有很多种,其中消息机制是一种常用的方式。线程消息机制是通过消息队列来实现线程之间的通信,它可以实现异步通信,线程不需要等待对方的响应,从而提高系统的并发性和响应速度。
下面是使用消息机制进行线程通信的基本步骤:
1. 创建消息队列:使用`msgget`函数创建一个消息队列,并返回一个消息队列标识符。
2. 发送消息:使用`msgsnd`函数向消息队列中发送消息,需要指定消息队列标识符、消息数据和消息长度等参数。
3. 接收消息:使用`msgrcv`函数从消息队列中接收消息,需要指定消息队列标识符、接收消息缓冲区和消息长度等参数。
4. 删除消息队列:使用`msgctl`函数删除消息队列,需要指定消息队列标识符和删除命令等参数。
下面是一个简单的示例代码,演示了如何使用消息机制在两个线程之间进行通信:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <pthread.h>
#include <sys/msg.h>
#define MAX_MSG_LEN 1024
struct msgbuf {
long mtype; // 消息类型
char mtext[MAX_MSG_LEN]; // 消息数据
};
void *thread_func(void *arg) {
int msqid;
struct msgbuf buf;
// 获取消息队列标识符
msqid = msgget((key_t)1234, 0666 | IPC_CREAT);
if (msqid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
// 接收消息
while (1) {
if (msgrcv(msqid, (void *)&buf, MAX_MSG_LEN, 1, 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", buf.mtext);
}
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t tid;
int msqid;
struct msgbuf buf;
// 创建线程
if (pthread_create(&tid, NULL, thread_func, NULL) != 0) {
perror("pthread_create failed");
exit(EXIT_FAILURE);
}
// 获取消息队列标识符
msqid = msgget((key_t)1234, 0666 | IPC_CREAT);
if (msqid == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
// 发送消息
strcpy(buf.mtext, "Hello, world!");
buf.mtype = 1;
if (msgsnd(msqid, (void *)&buf, strlen(buf.mtext) + 1, 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
// 等待线程结束
pthread_join(tid, NULL);
// 删除消息队列
if (msgctl(msqid, IPC_RMID, 0) == -1) {
perror("msgctl failed");
exit(EXIT_FAILURE);
}
return EXIT_SUCCESS;
}
```
在上面的示例中,主线程向消息队列中发送一条消息,子线程从消息队列中接收消息并打印。需要注意的是,消息类型必须大于0。如果需要多个不同类型的消息,可以使用不同的消息类型。
阅读全文
相关推荐
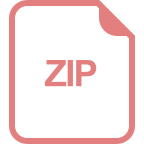
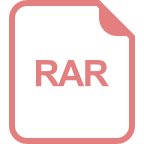
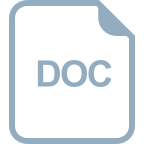

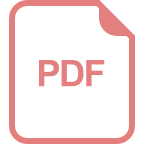
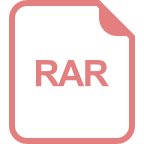
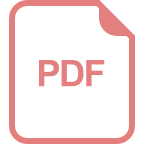
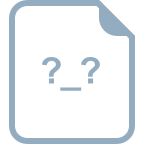
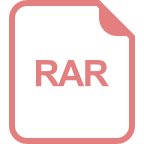
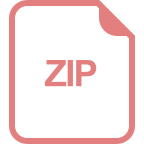
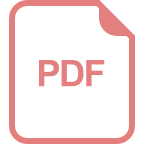
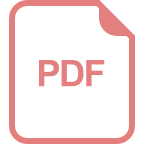
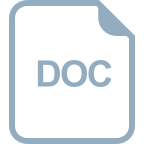
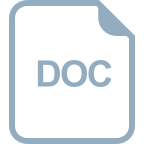

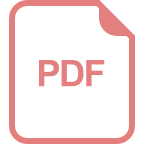
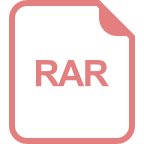
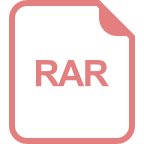
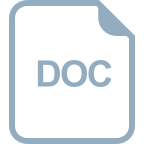