vue// 获取按钮和div元素const button = document.querySelector('button');const div = document.querySelector('div');// 添加点击事件监听器button.addEventListener('click', () => { // 修改div元素的background-color属性为红色 div.style.backgroundColor = 'red';});这段代码写在<script>标签里的哪
时间: 2024-01-22 13:19:35 浏览: 119
这段代码可以写在`<script>`标签中,或者单独放在一个`.js`文件中引入到HTML文件中。需要注意的是,这段代码应该放在HTML文件中对应的按钮和div元素之后,否则获取不到这两个元素。例如:
```html
<button>点击我</button>
<div>我会变成红色</div>
<script>
const button = document.querySelector('button');
const div = document.querySelector('div');
button.addEventListener('click', () => {
div.style.backgroundColor = 'red';
});
</script>
```
相关问题
const handleScroll = (index: number,e:Event) => { const container = document.querySelector(".index_"+index) as HTMLElement const scrollWidth = container.scrollWidth; // 滚动宽度 const scrollLeft = container.scrollLeft; // 滚动条的左侧距离容器左侧的距离 const clientWidth = container.clientWidth; // 可见宽度 if(scrollLeft >= (scrollWidth - clientWidth ) || (scrollWidth - clientWidth ) < 5){ console.log('最右边了',index) jumpToDetailPage(index) } } 这个滚动条事件应该用节流还是防抖,怎么写,vue3+TS项目中
这个滚动条事件应该使用节流,因为在用户快速滚动时,防抖会导致事件被多次触发,增加了性能开销。
在Vue3+TS项目中,你可以使用lodash库中的throttle函数来实现节流。具体可以按照以下步骤进行:
1. 在项目中安装lodash库:`npm install lodash`
2. 引入throttle函数:`import { throttle } from 'lodash'`
3. 在组件中定义一个变量来保存节流后的函数:
```
const handleScrollThrottled = throttle((index: number,e:Event) => {
const container = document.querySelector(".index_"+index) as HTMLElement
const scrollWidth = container.scrollWidth; // 滚动宽度
const scrollLeft = container.scrollLeft; // 滚动条的左侧距离容器左侧的距离
const clientWidth = container.clientWidth; // 可见宽度
if(scrollLeft >= (scrollWidth - clientWidth ) || (scrollWidth - clientWidth ) < 5){
console.log('最右边了',index)
jumpToDetailPage(index)
}
}, 200)
```
这里的throttle函数会将原函数的执行间隔限制为200ms。
4. 在滚动条事件中调用throttled函数:
```
const handleScroll = (index: number,e:Event) => {
handleScrollThrottled(index, e)
}
```
这样就完成了滚动条事件的节流处理。
vue document.querySelector获取动态的dom
在Vue.js中,`document.querySelector()` 是用于在文档中查找匹配指定CSS选择器的第一个元素的JavaScript DOM API。然而,它主要用于静态DOM操作,并不是直接针对Vue组件内的动态渲染。如果你想要在Vue中获取动态生成的DOM元素,你需要配合Vue提供的数据绑定、指令或者事件系统。
例如,假设你有一个动态生成的按钮:
```html
<button v-if="showButton" @click="handleClick">{{ buttonText }}</button>
```
在这个例子中,`v-if` 指令控制按钮是否显示,`@click` 挂载了一个点击事件处理器。如果你想在`handleClick`函数内部获取这个按钮,应该使用`this.$refs`属性,如果它是通过ref注册的:
```javascript
methods: {
handleClick() {
const button = this.$refs.myButton; // 假设按钮有ref="myButton"
if (button) {
// 现在你可以使用button元素了
console.log(button.innerText);
}
},
}
```
如果按钮是直接由模板动态创建的,你可以在事件处理函数中访问`$event.target`获取触发事件的元素。
阅读全文
相关推荐
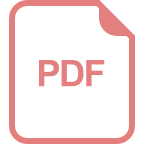
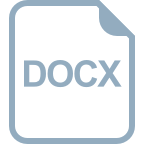
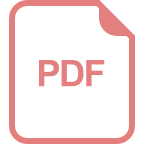













