优化代码 /// <summary> /// 获取验证码 /// </summary> /// <param name="phone"></param> /// <returns></returns> public ActionResult GetVerificationCode(string phone) { try { //if (phone.IsNullOrEmpty()) // return Error("未获取对应手机号,请刷新重试!"); ///生成六位随机码 var verifyCode = VerifyCode(); //存储临时验证码 SessionHelper.Session["verifyCode"] = verifyCode; string code = "{"code":""+ verifyCode + ""}"; //判断是否手机存在在系统重 if (phone.IsNullOrEmpty()) return Error("手机号码不允许为空"); //发送短信 //var result = PhoneMessage.PhoneMessageService("smsInvoice", "13003931757", verifyCode, 1, 0); var result = SmsHelper.SendSms(phone, "SMS_216730297", code); bindCustomerBusiness.GetLogger().WriteOperateLogNoUser("获取验证码", result.ToJson(), true); return Success(); } catch (Exception ex) { return Error(ex.StackTrace); } } public ActionResult GetVerificationCode2(string phone) { try { //if (phone.IsNullOrEmpty()) // return Error("未获取对应手机号,请刷新重试!"); ///生成六位随机码 var verifyCode = VerifyCode(); //存储临时验证码 SessionHelper.Session["verifyCode"] = verifyCode; string code = "{"code":"" + verifyCode + ""}"; //判断是否手机存在在系统重 if (phone.IsNullOrEmpty()) return Error("手机号码不允许为空"); else { var phoneCount = bindCustomerBusiness.Service.GetIQueryable<Base_User>(). Where(a => a.Phone == phone).Count(); if (phoneCount == 0) return Error("手机号未绑定,首次应扫码登录"); } //发送短信 //var result = PhoneMessage.PhoneMessageService("smsInvoice", "13003931757", verifyCode, 1, 0); var result = SmsHelper.SendSms(phone, "SMS_216730297", code); bindCustomerBusiness.GetLogger().WriteOperateLogNoUser("获取验证码", result.ToJson(), true); return Success(); } catch (Exception ex) { return Error(ex.StackTrace); } }
时间: 2024-04-26 21:26:34 浏览: 84
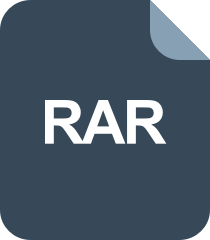
param.rar_Linux/Unix编程_Unix_Linux_
这段代码可以进行如下的优化:
1. 将相同的代码封装成一个方法,避免代码重复。
2. 将短信模板ID和验证码的格式放到配置文件中,方便维护。
3. 使用参数校验框架,比如 FluentValidation,来校验手机号码是否为空。
4. 将异常信息记录到日志中,方便问题排查。
下面是优化后的代码:
```
/// <summary>
/// 获取验证码
/// </summary>
/// <param name="phone"></param>
/// <param name="bindFirstTime"></param>
/// <returns></returns>
public ActionResult GetVerificationCode(string phone, bool bindFirstTime = false)
{
try
{
// 参数校验
var validator = new GetVerificationCodeValidator();
var validationResult = validator.Validate(new GetVerificationCodeInput { Phone = phone });
if (!validationResult.IsValid)
{
return Error(validationResult.Errors.First().ErrorMessage);
}
// 生成六位随机码
var verifyCode = VerifyCode();
// 存储临时验证码
SessionHelper.Session["verifyCode"] = verifyCode;
// 判断是否首次绑定
if (bindFirstTime)
{
var phoneCount = bindCustomerBusiness.Service.GetIQueryable<Base_User>().Where(a => a.Phone == phone).Count();
if (phoneCount == 0)
{
return Error("手机号未绑定,首次应扫码登录");
}
}
// 发送短信
var smsTemplateId = ConfigurationManager.AppSettings["SmsTemplateId"];
var smsContent = string.Format(ConfigurationManager.AppSettings["SmsContentFormat"], verifyCode);
var result = SmsHelper.SendSms(phone, smsTemplateId, smsContent);
// 记录日志
bindCustomerBusiness.GetLogger().WriteOperateLogNoUser("获取验证码", result.ToJson(), true);
return Success();
}
catch (Exception ex)
{
// 记录异常日志
bindCustomerBusiness.GetLogger().WriteErrorLog(ex.Message, ex.StackTrace);
return Error("获取验证码失败");
}
}
public class GetVerificationCodeValidator : AbstractValidator<GetVerificationCodeInput>
{
public GetVerificationCodeValidator()
{
RuleFor(x => x.Phone).NotEmpty().WithMessage("手机号码不能为空");
}
}
public class GetVerificationCodeInput
{
public string Phone { get; set; }
}
```
阅读全文
相关推荐
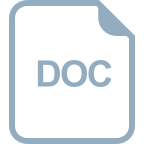
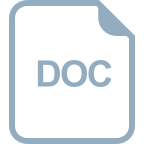
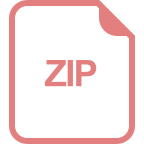
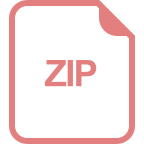
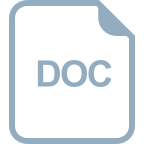
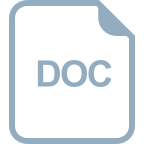
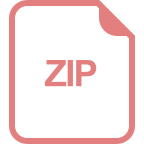
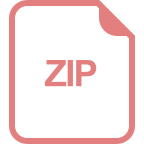
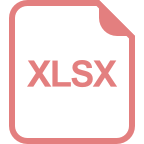
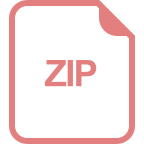
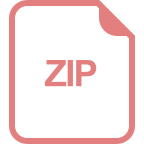
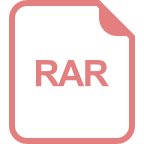
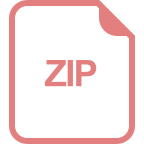
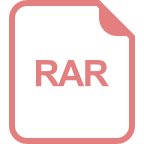