不使用LINQ查询和操作集合 改进代码 namespace SandwichCalories { class Program { static void Main(string[] args) { // sandwich ingredients and their associated calories Dictionary<string, int> ingredients = new Dictionary<string, int>() { { "Bread", 66 }, { "Ham", 72 }, { "Bologna", 57 }, { "Chicken", 17 }, { "Corned Beef", 53 }, { "Salami", 40 }, { "Cheese, American", 104 }, { "Cheese, Cheddar", 113 }, { "Cheese, Havarti", 105 }, { "Mayonnaise", 94 }, { "Mustard", 10 }, { "Butter", 102 }, { "Garlic Aioli", 100 }, { "Sriracha", 15 }, { "Dressing, Ranch", 73 }, { "Dressing, 1000 Island", 59 }, { "Lettuce", 5 }, { "Tomato", 4 }, { "Cucumber", 4 }, { "Banana Pepper", 10 }, { "Green Pepper", 3 }, { "Red Onion", 6 }, { "Spinach", 7 }, { "Avocado", 64 } }; // prompt user for calorie range Console.Write("Enter minimum calories: "); int min_calories = int.Parse(Console.ReadLine()); Console.Write("Enter maximum calories: "); int max_calories = int.Parse(Console.ReadLine()); // calculate the minimum and maximum calories for the sandwich int min_sandwich_calories = 2 * ingredients["Bread"] + ingredients.Values.Min() * 2; int max_sandwich_calories = 2 * ingredients["Bread"] + ingredients.Values.Max() * 2; // check if the calorie range is valid if (max_calories < min_sandwich_calories) { Console.WriteLine("Sorry, it is impossible to create a sandwich within the given calorie range."); } else { // create the sandwich List<string> sandwich = new List<string> { "Bread" }; int sandwich_calories = 1 * ingredients["Bread"]; while (sandwich_calories < min_calories) { // add random ingredient string ingredient = ingredients.Keys.ElementAt(new Random().Next(ingredients.Count)); sandwich.Add(ingredient); sandwich_calories += ingredients[ingredient]; } while (sandwich_calories <= max_calories) { // add random ingredient string ingredient = ingredients.Keys.ElementAt(new Random().Next(ingredients.Count)); // check if the ingredient is the same as the previous one if (sandwich.Count >= 3 && ingredient == sandwich[sandwich.Count - 2]) { continue; } sandwich.Add(ingredient); sandwich_calories += ingredients[ingredient]; // check if the sandwich is already at the maximum calorie limit if (sandwich_calories == max_sandwich_calories) { break; } } // add the last slice of bread sandwich.Add("Bread"); // print the sandwich and its total calories Console.WriteLine("Your sandwich: " + string.Join(", ", sandwich)); Console.WriteLine("Total calories: " + sandwich_calories); } } } }
时间: 2023-07-19 14:41:22 浏览: 89
以下是不使用LINQ查询和操作集合改进的代码,这里使用了foreach循环和if条件语句来实现相同的功能:
```csharp
using System;
using System.Collections.Generic;
namespace SandwichCalories
{
class Program
{
static void Main(string[] args)
{
// sandwich ingredients and their associated calories
Dictionary<string, int> ingredients = new Dictionary<string, int>() {
{ "Bread", 66 },
{ "Ham", 72 },
{ "Bologna", 57 },
{ "Chicken", 17 },
{ "Corned Beef", 53 },
{ "Salami", 40 },
{ "Cheese, American", 104 },
{ "Cheese, Cheddar", 113 },
{ "Cheese, Havarti", 105 },
{ "Mayonnaise", 94 },
{ "Mustard", 10 },
{ "Butter", 102 },
{ "Garlic Aioli", 100 },
{ "Sriracha", 15 },
{ "Dressing, Ranch", 73 },
{ "Dressing, 1000 Island", 59 },
{ "Lettuce", 5 },
{ "Tomato", 4 },
{ "Cucumber", 4 },
{ "Banana Pepper", 10 },
{ "Green Pepper", 3 },
{ "Red Onion", 6 },
{ "Spinach", 7 },
{ "Avocado", 64 }
};
// prompt user for calorie range
Console.Write("Enter minimum calories: ");
int min_calories = int.Parse(Console.ReadLine());
Console.Write("Enter maximum calories: ");
int max_calories = int.Parse(Console.ReadLine());
// calculate the minimum and maximum calories for the sandwich
int min_sandwich_calories = 2 * ingredients["Bread"] + GetMinValue(ingredients.Values) * 2;
int max_sandwich_calories = 2 * ingredients["Bread"] + GetMaxValue(ingredients.Values) * 2;
// check if the calorie range is valid
if (max_calories < min_sandwich_calories)
{
Console.WriteLine("Sorry, it is impossible to create a sandwich within the given calorie range.");
}
else
{
// create the sandwich
List<string> sandwich = new List<string> { "Bread" };
int sandwich_calories = 1 * ingredients["Bread"];
while (sandwich_calories < min_calories)
{
// add random ingredient
string ingredient = GetRandomIngredient(ingredients);
sandwich.Add(ingredient);
sandwich_calories += ingredients[ingredient];
}
while (sandwich_calories <= max_calories)
{
// add random ingredient
string ingredient = GetRandomIngredient(ingredients);
// check if the ingredient is the same as the previous one
if (sandwich.Count >= 3 && ingredient == sandwich[sandwich.Count - 2])
{
continue;
}
sandwich.Add(ingredient);
sandwich_calories += ingredients[ingredient];
// check if the sandwich is already at the maximum calorie limit
if (sandwich_calories == max_sandwich_calories)
{
break;
}
}
// add the last slice of bread
sandwich.Add("Bread");
// print the sandwich and its total calories
Console.WriteLine("Your sandwich: " + string.Join(", ", sandwich));
Console.WriteLine("Total calories: " + sandwich_calories);
}
}
// helper methods
static int GetMinValue(IEnumerable<int> values)
{
int minValue = int.MaxValue;
foreach (int value in values)
{
if (value < minValue)
{
minValue = value;
}
}
return minValue;
}
static int GetMaxValue(IEnumerable<int> values)
{
int maxValue = int.MinValue;
foreach (int value in values)
{
if (value > maxValue)
{
maxValue = value;
}
}
return maxValue;
}
static string GetRandomIngredient(Dictionary<string, int> ingredients)
{
int index = new Random().Next(ingredients.Count);
int i = 0;
foreach (string key in ingredients.Keys)
{
if (i == index)
{
return key;
}
i++;
}
return null;
}
}
}
```
在这个改进的代码中,我们使用了GetMinValue和GetMaxValue方法来获取字典中值的最小值和最大值,使用GetRandomIngredient方法来获取随机的食材名称。虽然这种方式代码量增加了,但是这种方式可以更好地理解代码的每个步骤,也可以更好地掌控代码的细节。
阅读全文
相关推荐











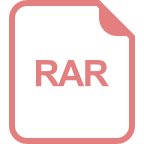






