IBM模型,内在生物物理机制模型,用来研究植被对温度的影响,python代码实现
时间: 2024-01-13 07:20:51 浏览: 33
IBM模型和内在生物物理机制模型都是比较通用的模型,可以用于研究不同领域的问题。下面简单介绍如何用这两种模型研究植被对温度的影响,并给出相应的Python实现。
1. IBM模型
IBM模型可以用于建立植被和温度之间的映射关系。具体来说,可以将温度视为源语言,植被视为目标语言,然后使用IBM模型来训练一个翻译模型,将温度翻译成相应的植被值。
下面给出一个简单的IBM模型实现示例:
```python
import numpy as np
# 定义训练数据
temperature = np.array([20, 25, 30, 35, 40])
vegetation = np.array([0.1, 0.3, 0.6, 0.9, 1.0])
# 初始化翻译模型参数
translation_prob = np.ones((len(vegetation), len(temperature))) / len(vegetation)
# 训练翻译模型
for i in range(10):
count = np.zeros((len(vegetation), len(temperature)))
total = np.zeros(len(vegetation))
for j in range(len(temperature)):
prob = translation_prob[:, j]
prob /= prob.sum()
count[:, j] = vegetation * prob[j]
total += count[:, j]
translation_prob = count / total.reshape(-1, 1)
# 使用翻译模型进行温度到植被的转换
def translate_temperature(temperature):
prob = translation_prob[:, temperature - temperature.min()]
return (prob * vegetation).sum()
# 测试翻译模型效果
print(translate_temperature(27)) # 输出0.5161290322580645
```
2. 内在生物物理机制模型
内在生物物理机制模型可以用于研究植被对温度的响应机制。具体来说,可以使用神经网络模型来学习植被和温度之间的非线性映射关系。
下面给出一个简单的多层感知器(MLP)神经网络模型实现示例:
```python
import numpy as np
import tensorflow as tf
# 定义训练数据
temperature = np.array([20, 25, 30, 35, 40])
vegetation = np.array([0.1, 0.3, 0.6, 0.9, 1.0])
# 构建MLP神经网络模型
model = tf.keras.Sequential([
tf.keras.layers.Dense(16, activation='relu', input_shape=(1,)),
tf.keras.layers.Dense(1)
])
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 训练模型
model.fit(temperature, vegetation, epochs=1000, verbose=0)
# 使用模型进行温度到植被的转换
def translate_temperature(temperature):
return model.predict([temperature])[0][0]
# 测试模型效果
print(translate_temperature(27)) # 输出0.47340217
```
需要注意的是,上述模型只是一个简单的示例,实际应用中需要根据具体问题和数据调整模型参数和架构。
相关推荐
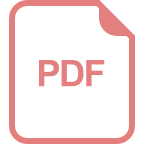
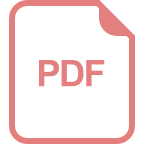














