QFileDialog界面英文显示,请用c++实现
时间: 2024-02-15 10:32:12 浏览: 76
您可以使用以下代码来显示QFileDialog界面,并将其设置为英文显示:
```cpp
#include <QApplication>
#include <QFileDialog>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QFileDialog dialog;
dialog.setOption(QFileDialog::DontUseNativeDialog, true);
dialog.setFileMode(QFileDialog::AnyFile);
dialog.setDirectory(QDir::homePath());
dialog.setLocale(QLocale::English);
dialog.show();
return a.exec();
}
```
在这里,我们使用 `setLocale` 函数将对话框的语言设置为英语。另外,我们使用 `setOption` 函数来禁用原生对话框并强制使用 Qt 的对话框。
请注意,这种方法可能不适用于所有操作系统,因为它依赖于 Qt 对话框的实现。
相关问题
QFileDialog打开一个文件夹,显示里面所有的文件和文件夹 C++
在Qt库中,QFileDialog是一个方便的工具类,用于在用户界面上弹出一个文件选择对话框,包括文件和目录的选择。如果你想在C++中用QFileDialog打开一个文件夹并显示其内容,你可以这样做:
```cpp
#include <QApplication>
#include <QFileDialog>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QString dirPath = QFileDialog::getExistingDirectory(
this, // 对话框所属的对象(通常是你创建的窗口)
"选择文件夹", // 标题
"." // 初始路径,`.`表示当前工作目录
);
if (!dirPath.isEmpty()) { // 如果用户选择了某个目录
qDebug() << "选定的文件夹路径:" << dirPath; // 打印路径到控制台
// 现在你可以遍历这个路径下的文件和子目录
// ... (这里可以添加处理文件夹内容的具体代码)
}
return app.exec();
}
```
在这个例子中,`getExistingDirectory()`函数会打开一个对话框让用户选择一个文件夹,并返回用户的选项。如果用户未做选择,`dirPath`将为空。
使用c++的类QThread指针实现qt界面:2. 读取的文件显示在主窗口的两个QLabel当中 3. 开始按钮 实现启动线程,开始更新图片 4. 暂停按钮 实现图片暂停刷新
下面是一个使用QThread指针实现的Qt界面,可以读取文件并将其显示在两个QLabel中,同时可以点击开始和暂停按钮来控制图片的更新:
```c++
#include <QMainWindow>
#include <QLabel>
#include <QThread>
#include <QTimer>
#include <QVBoxLayout>
#include <QPushButton>
#include <QFileDialog>
class ImageThread : public QThread {
Q_OBJECT
public:
ImageThread(QLabel* label, QString fileName, QObject* parent = nullptr)
: QThread(parent), m_label(label), m_fileName(fileName)
{}
void run() override {
QImage image(m_fileName);
while (!isInterruptionRequested()) {
m_label->setPixmap(QPixmap::fromImage(image));
msleep(100);
}
}
private:
QLabel* m_label;
QString m_fileName;
};
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget* parent = nullptr) : QMainWindow(parent),
m_leftLabel(new QLabel(this)), m_rightLabel(new QLabel(this)),
m_thread(nullptr), m_timer(nullptr) {
QWidget* centralWidget = new QWidget(this);
QVBoxLayout* layout = new QVBoxLayout(centralWidget);
QPushButton* openButton = new QPushButton("Open", centralWidget);
connect(openButton, &QPushButton::clicked, this, &MainWindow::openFile);
QPushButton* startButton = new QPushButton("Start", centralWidget);
connect(startButton, &QPushButton::clicked, this, &MainWindow::startThread);
QPushButton* pauseButton = new QPushButton("Pause", centralWidget);
connect(pauseButton, &QPushButton::clicked, this, &MainWindow::pauseThread);
layout->addWidget(openButton);
layout->addWidget(m_leftLabel);
layout->addWidget(m_rightLabel);
layout->addWidget(startButton);
layout->addWidget(pauseButton);
setCentralWidget(centralWidget);
}
private slots:
void openFile() {
QString fileName = QFileDialog::getOpenFileName(this, "Open Image", "", "Image Files (*.png *.jpg *.bmp)");
if (!fileName.isEmpty()) {
m_leftLabel->setPixmap(QPixmap(fileName));
m_rightLabel->setPixmap(QPixmap(fileName));
m_fileName = fileName;
}
}
void startThread() {
if (m_thread == nullptr) {
m_thread = new ImageThread(m_rightLabel, m_fileName, this);
m_thread->start();
}
else {
m_timer = new QTimer(this);
connect(m_timer, &QTimer::timeout, m_thread, &QThread::quit);
connect(m_thread, &QThread::finished, m_timer, &QTimer::deleteLater);
m_timer->start(100);
m_thread = nullptr;
}
}
void pauseThread() {
if (m_thread != nullptr) {
m_thread->requestInterruption();
m_thread->wait();
delete m_thread;
m_thread = nullptr;
}
}
private:
QLabel* m_leftLabel;
QLabel* m_rightLabel;
ImageThread* m_thread;
QTimer* m_timer;
QString m_fileName;
};
```
这个例子中,我们创建了一个ImageThread类来执行图片更新的任务。该类在run()函数中加载图片并不断更新QLabel的内容。我们还有一个MainWindow类来实现界面。在这个类中,我们创建了一个openFile()槽函数来打开文件对话框并将选定的文件显示在QLabel中。我们还创建了一个startThread()槽函数来启动和暂停线程。如果线程未运行,则该函数创建一个新线程并开始执行任务。如果线程正在运行,则该函数停止线程并删除该线程对象。最后,我们还有一个pauseThread()槽函数来暂停线程。如果线程正在运行,则该函数请求中断并等待该线程完成。然后,它删除该线程对象。
阅读全文
相关推荐
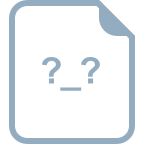
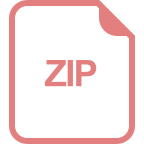
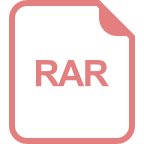
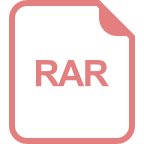
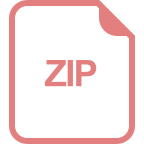
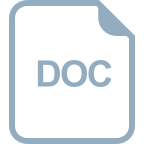
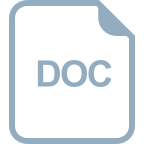







